Java 批量打印二维码
时间: 2023-08-29 21:05:34 浏览: 95
要在 Java 中批量打印二维码,可以使用 Zebra 打印机和 ZPL 程序设计语言。以下是一个简单的示例程序,可以将多个二维码一次性打印到 Zebra 打印机上。
```java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class BatchPrintQR {
public static void main(String[] args) {
try {
// 读取二维码图片文件夹下的所有文件
Path dirPath = Paths.get("C:/qrcodes");
List<Path> files = Files.list(dirPath).toList();
// 打印机连接字符串
String printerConnectionString = "tcp://192.168.1.100:9100";
// 遍历所有文件,生成 ZPL 命令并发送到打印机
for (int i = 0; i < files.size(); i++) {
Path filePath = files.get(i);
byte[] fileBytes = Files.readAllBytes(filePath);
String zplCommand = "^XA^FO50,50^BQN,2,10^FDMA," + i + "^FS^XZ";
ZebraPrinter.sendZplCommand(printerConnectionString, zplCommand, fileBytes);
}
System.out.println("All QR Codes have been printed!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
class ZebraPrinter {
public static void sendZplCommand(String printerConnectionString, String zplCommand, byte[] imageData) {
try {
// 打印机连接
Connection connection = new TcpConnection(printerConnectionString);
connection.open();
// 发送 ZPL 命令
connection.write(zplCommand.getBytes());
// 发送图片数据
if (imageData != null) {
String imageStartCommand = "^GFA,";
imageStartCommand += imageData.length + ",";
imageStartCommand += imageData.length + ",";
imageStartCommand += "0,"; // 图片类型,0 表示黑白
imageStartCommand += " ";
connection.write(imageStartCommand.getBytes());
connection.write(imageData);
}
// 断开连接
connection.close();
} catch (ConnectionException e) {
e.printStackTrace();
} catch (ZebraPrinterLanguageUnknownException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个程序假设所有的二维码图片都保存在 C:/qrcodes 文件夹下。程序会读取该文件夹下的所有文件,并将每个文件生成一个 ZPL 命令发送到打印机。ZPL 命令中包含了二维码图片的位置和编号,打印机会将所有的二维码一次性打印出来。
阅读全文
相关推荐
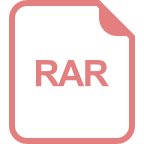
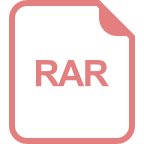
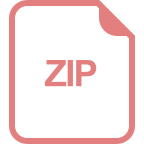
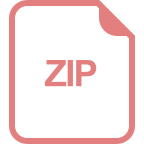
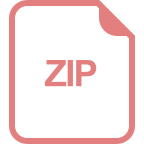
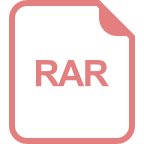
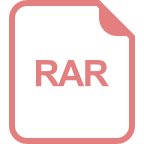
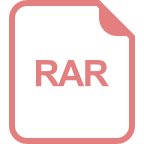
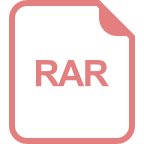
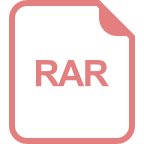
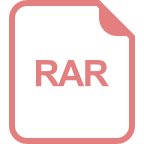
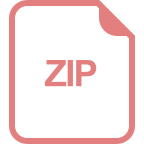
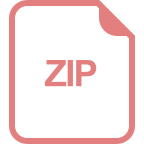
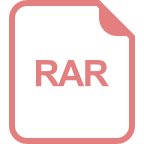
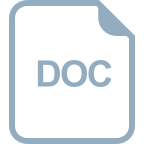
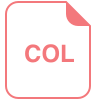
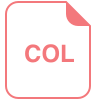