public class FileSplitter { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("请输入被分割的大文件的路径:"); String sourceFilePath = scanner.nextLine(); System.out.print("请输入分割块大小(单位MB):"); int blockSize = scanner.nextInt() * 1024 * 1024; scanner.nextLine(); // 消耗换行符 System.out.print("请输入分割后小文件存放的路径:"); String destinationPath = scanner.nextLine(); try { File sourceFile = new File(sourceFilePath); // if (!sourceFile.exists()) { // System.out.println("文件不存在!"); // return; // } long fileSize = sourceFile.length(); int numberOfParts = (int) Math.ceil((double) fileSize / blockSize); try (FileChannel sourceChannel = FileChannel.open(Paths.get(sourceFilePath), StandardOpenOption.READ)) { for (int i = 0; i < numberOfParts; i++) { String partFileName = destinationPath + File.separator + i + ".part"; try (FileChannel destinationChannel = FileChannel.open(Paths.get(partFileName), StandardOpenOption.CREATE, StandardOpenOption.WRITE)) { long position = i * blockSize; long size = Math.min(blockSize, fileSize - position); sourceChannel.transferTo(position, size, destinationChannel); } System.out.println("已生成文件:" + partFileName); } } } catch (IOException e) { e.printStackTrace(); } } }分析一下这段代码
时间: 2024-02-15 21:28:24 浏览: 72
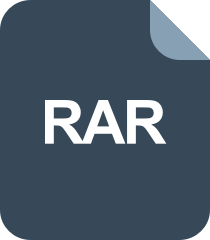
FileSplitter大文件切割
这段代码实现了一个文件分割器,可以将一个大文件分割成多个小文件。主要的实现是通过使用 Java NIO 中的 FileChannel 和 transferTo 方法来实现文件的读写和分割。具体实现步骤如下:
1. 从控制台读取用户输入的被分割文件的路径、分割块大小和分割后小文件存放的路径。
2. 计算需要分割成多少个小文件。
3. 打开被分割文件对应的 FileChannel,并循环生成小文件,每个小文件大小为指定的分割块大小,直到被分割文件全部读取。
4. 在循环中,通过 transferTo 方法将被分割文件的指定位置开始的指定大小的数据写入到对应的小文件的 FileChannel 中。
5. 输出已生成的小文件名。
需要注意的是,这段代码中的一些注释被注释掉了,需要根据实际情况进行判断和使用。另外,对于大文件的分割,还需要考虑一些性能和安全方面的问题,这里没有进行详细的讨论。
阅读全文
相关推荐
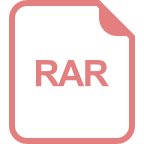
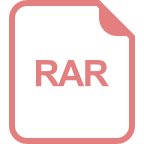
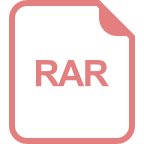
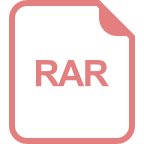
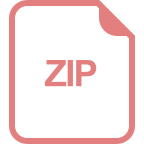
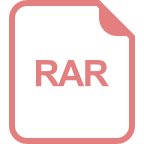
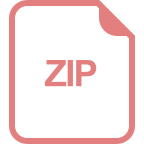
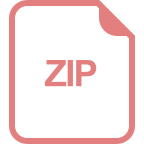
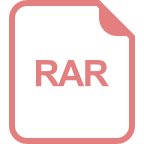
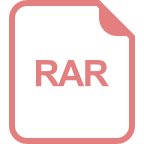
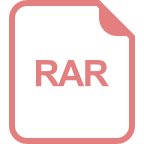

