编写可以实现学生成绩简单管理系统。本系统主要实现以下功能:(1)录入学生信息。(2)按学号查询学生信息。(3)按姓名查询学生信息。(4)添加一个学生信息。(5)按学号删除学生信息(6)按学号修改学生信息(7)显示所有学生信息(8)按课程排序(有高到底)输出显示学生信息(9)按分数段统计学生信息(10)退出系统
时间: 2023-12-01 21:02:46 浏览: 39
好的,我可以为您提供一份 Python 代码,实现上述的学生成绩简单管理系统。请您先创建一个名为 `students.txt` 的空文本文件,用于存储学生信息。
```python
import os
def main():
while True:
print('''
=============学生成绩管理系统=============
1. 录入学生信息
2. 按学号查询学生信息
3. 按姓名查询学生信息
4. 添加一个学生信息
5. 按学号删除学生信息
6. 按学号修改学生信息
7. 显示所有学生信息
8. 按课程排序输出显示学生信息
9. 按分数段统计学生信息
0. 退出系统
==========================================
''')
choice = input('请输入对应的数字:')
if choice == '1':
add_student()
elif choice == '2':
search_by_id()
elif choice == '3':
search_by_name()
elif choice == '4':
add_one_student()
elif choice == '5':
delete_by_id()
elif choice == '6':
modify_by_id()
elif choice == '7':
show_all_students()
elif choice == '8':
sort_by_course()
elif choice == '9':
statistics_by_score()
elif choice == '0':
break
else:
print('输入有误,请重新输入!')
def add_student():
try:
with open('students.txt', 'a') as file:
while True:
id = input('请输入学号(输入0返回上一级):')
if id != '0':
name = input('请输入姓名:')
math = input('请输入数学成绩:')
english = input('请输入英语成绩:')
programming = input('请输入编程成绩:')
file.write(id + ' ' + name + ' ' + math + ' ' + english + ' ' + programming + '\n')
else:
break
except Exception as e:
print('出现异常:', e)
def search_by_id():
try:
id = input('请输入要查询的学生学号:')
with open('students.txt', 'r') as file:
for line in file:
student = line.strip().split()
if student[0] == id:
print('学号\t姓名\t数学\t英语\t编程')
print(line)
break
else:
print('没有找到该学生!')
except Exception as e:
print('出现异常:', e)
def search_by_name():
try:
name = input('请输入要查询的学生姓名:')
with open('students.txt', 'r') as file:
flag = False
for line in file:
student = line.strip().split()
if student[1] == name:
if not flag:
print('学号\t姓名\t数学\t英语\t编程')
print(line)
flag = True
if not flag:
print('没有找到该学生!')
except Exception as e:
print('出现异常:', e)
def add_one_student():
try:
id = input('请输入学号:')
name = input('请输入姓名:')
math = input('请输入数学成绩:')
english = input('请输入英语成绩:')
programming = input('请输入编程成绩:')
with open('students.txt', 'a') as file:
file.write(id + ' ' + name + ' ' + math + ' ' + english + ' ' + programming + '\n')
print('添加成功!')
except Exception as e:
print('出现异常:', e)
def delete_by_id():
try:
id = input('请输入要删除的学生学号:')
with open('students.txt', 'r') as rfile:
students = rfile.readlines()
with open('students.txt', 'w') as wfile:
flag = False
for student in students:
if student.split()[0] != id:
wfile.write(student)
else:
print('删除成功!')
flag = True
if not flag:
print('没有找到该学生!')
except Exception as e:
print('出现异常:', e)
def modify_by_id():
try:
id = input('请输入要修改的学生学号:')
with open('students.txt', 'r') as rfile:
students = rfile.readlines()
with open('students.txt', 'w') as wfile:
flag = False
for student in students:
if student.split()[0] == id:
name = input('请输入姓名:')
math = input('请输入数学成绩:')
english = input('请输入英语成绩:')
programming = input('请输入编程成绩:')
student = id + ' ' + name + ' ' + math + ' ' + english + ' ' + programming + '\n'
flag = True
wfile.write(student)
if flag:
print('修改成功!')
else:
print('没有找到该学生!')
except Exception as e:
print('出现异常:', e)
def show_all_students():
try:
with open('students.txt', 'r') as file:
print('学号\t姓名\t数学\t英语\t编程')
for line in file:
print(line, end='')
except Exception as e:
print('出现异常:', e)
def sort_by_course():
try:
with open('students.txt', 'r') as file:
students = file.readlines()
students.sort(key=lambda x: int(x.strip().split()[3])+int(x.strip().split()[4])+int(x.strip().split()[5]), reverse=True)
print('学号\t姓名\t数学\t英语\t编程')
for student in students:
print(student, end='')
except Exception as e:
print('出现异常:', e)
def statistics_by_score():
try:
min_score = int(input('请输入最低分数:'))
max_score = int(input('请输入最高分数:'))
with open('students.txt', 'r') as file:
count = 0
for line in file:
student = line.strip().split()
score = int(student[2]) + int(student[3]) + int(student[4])
if min_score <= score <= max_score:
count += 1
print(f'总共有 {count} 个学生在 {min_score} 到 {max_score} 分之间!')
except Exception as e:
print('出现异常:', e)
if __name__ == '__main__':
main()
```
在运行程序之前,需要确保 Python 环境已经安装,同时请将以上代码复制到一个名为 `students.py` 的文件中。在终端中执行 `python students.py` 即可运行程序。
相关推荐
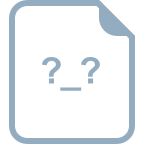
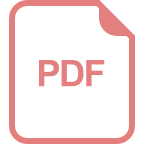
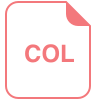
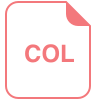
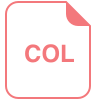
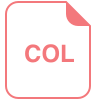
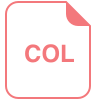









