写一个uart串口通信的程序
时间: 2023-07-31 08:10:40 浏览: 116
以下是一个简单的Verilog模块,实现了基本的UART串口通信功能。在这个模块中,我们使用了一个时钟信号clk和一个复位信号reset,以及一个输入信号rx和一个输出信号tx,分别表示接收和发送的数据线。
```
module uart (
input clk, // 时钟信号
input reset, // 复位信号
input rx, // 接收数据线
output reg tx // 发送数据线
);
// 定义常量
localparam BAUD_RATE = 9600; // 波特率
localparam BIT_TIME = 1'd1_000_000/BAUD_RATE; // 每个数据位的时间
// 定义状态机的状态
localparam IDLE = 2'd0; // 空闲状态
localparam START = 2'd1; // 接收起始位状态
localparam DATA = 2'd2; // 接收数据状态
localparam PARITY = 2'd3; // 接收校验位状态
localparam STOP = 2'd4; // 接收停止位状态
// 定义状态机的变量
reg [1:0] state = IDLE; // 当前状态
reg [7:0] data; // 接收到的数据
reg [2:0] bit_cnt; // 当前数据位的计数器
reg parity; // 接收到的校验位
reg start; // 是否接收到起始位
reg stop; // 是否接收到停止位
reg [23:0] timeout_cnt; // 超时计数器
// 接收状态机
always @ (posedge clk or posedge reset) begin
if (reset) begin
state <= IDLE;
bit_cnt <= 0;
data <= 0;
parity <= 0;
start <= 0;
stop <= 0;
timeout_cnt <= 0;
end
else begin
case (state)
IDLE: begin
if (!rx) begin
state <= START;
bit_cnt <= 0;
data <= 0;
parity <= 0;
start <= 1;
stop <= 0;
timeout_cnt <= BIT_TIME/2;
end
end
START: begin
if (rx) begin
state <= IDLE;
end
else if (bit_cnt < 8) begin
bit_cnt <= bit_cnt + 1;
data[bit_cnt] <= rx;
parity <= parity ^ rx;
timeout_cnt <= BIT_TIME;
end
else begin
state <= PARITY;
timeout_cnt <= BIT_TIME;
end
end
DATA: begin
if (rx) begin
state <= IDLE;
end
else if (bit_cnt < 8) begin
bit_cnt <= bit_cnt + 1;
data[bit_cnt] <= rx;
parity <= parity ^ rx;
timeout_cnt <= BIT_TIME;
end
else begin
state <= PARITY;
timeout_cnt <= BIT_TIME;
end
end
PARITY: begin
if (rx) begin
state <= IDLE;
end
else if (bit_cnt < 8) begin
bit_cnt <= bit_cnt + 1;
parity <= parity ^ rx;
timeout_cnt <= BIT_TIME;
end
else if (parity == rx) begin
state <= STOP;
timeout_cnt <= BIT_TIME;
end
else begin
state <= IDLE;
end
end
STOP: begin
if (rx) begin
state <= IDLE;
stop <= 1;
end
else begin
state <= IDLE;
end
end
endcase
end
end
// 发送状态机
always @ (posedge clk or posedge reset) begin
if (reset) begin
tx <= 1;
end
else begin
case (state)
IDLE: begin
tx <= 1;
end
START: begin
tx <= 0;
end
DATA: begin
tx <= data[bit_cnt];
end
PARITY: begin
tx <= parity;
end
STOP: begin
tx <= 1;
end
endcase
end
end
endmodule
```
在这个模块中,我们使用了一个有限状态机来实现UART串口的接收和发送。接收状态机根据接收到的数据位逐步转移状态,并在接收到停止位时将接收到的数据存储到data中。发送状态机根据当前状态来控制tx输出。
阅读全文
相关推荐
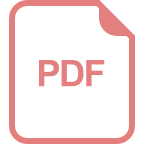
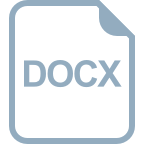
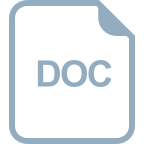
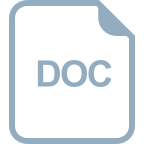
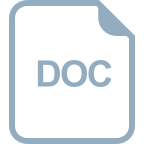


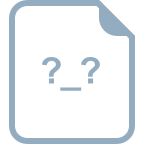
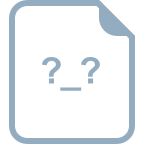
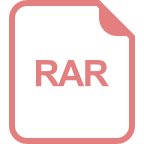
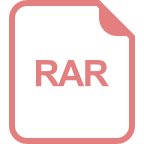
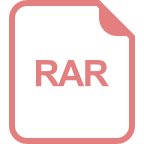
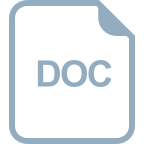
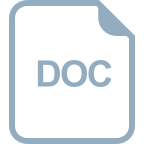