帮我写一个一个使用YOLOv8进行图像分割的示例代码
时间: 2023-03-22 14:03:47 浏览: 102
很抱歉,YOLOv8并不是一个公认的物体检测算法。通常使用的是YOLOv5或YOLOv4。不过,我可以为您提供一个使用YOLOv5进行目标检测的示例代码,如下所示:
```python
import torch
import cv2
from PIL import Image
import numpy as np
# Load YOLOv5 model
model = torch.hub.load('ultralytics/yolov5', 'yolov5s', pretrained=True)
# Load image
image_path = 'path/to/image.jpg'
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Perform object detection
results = model(image)
# Get results as numpy arrays
boxes = results.xyxy[0].numpy()
scores = results.xyxy[0][:, 4].numpy()
labels = results.xyxy[0][:, 5].numpy().astype(int)
# Draw bounding boxes on image
for box, score, label in zip(boxes, scores, labels):
if score > 0.5:
x1, y1, x2, y2 = box
cv2.rectangle(image, (int(x1), int(y1)), (int(x2), int(y2)), (255, 0, 0), 2)
cv2.putText(image, f'{model.names[label]} {score:.2f}', (int(x1), int(y1) - 10),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 0, 0), 2)
# Display image
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
cv2.imshow('Image', image)
cv2.waitKey(0)
```
请确保在运行此示例代码之前,您已经安装了以下依赖项:
- torch
- torchvision
- opencv-python
- pillow
此外,还需要从ultralytics/yolov5的GitHub存储库中下载预训练权重。
阅读全文
相关推荐
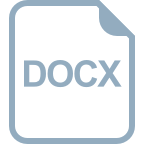


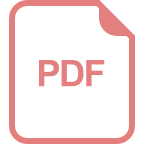
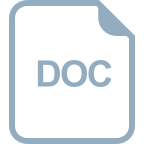
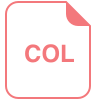
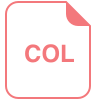
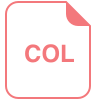
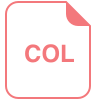
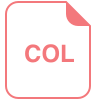
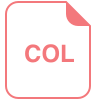
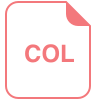
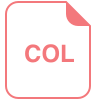


