C语言编写输入一个英文字符串,将所有大写字符转成小写,所有小写字符转成大写
时间: 2024-02-16 10:13:45 浏览: 25
好的,这个问题很简单。可以使用 C 语言中的字符处理函数 `toupper()` 和 `tolower()` 来实现。具体步骤如下:
1. 读入一个英文字符串,可以使用 `scanf()` 或 `gets()` 函数;
2. 循环遍历字符串中的每个字符;
3. 如果字符是大写字母,就调用 `tolower()` 函数将其转为小写字母;
4. 如果字符是小写字母,就调用 `toupper()` 函数将其转为大写字母;
5. 最后将处理后的字符串输出即可。
以下是示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i;
printf("请输入一个英文字符串:");
scanf("%s", str);
for (i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
str[i] = tolower(str[i]);
} else if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
希望能够解答您的问题!
相关问题
C语言输入一个字符串将其中大写的字母转成小写,将小写的字母转大写
可以使用C语言中的字符函数`tolower()`和`toupper()`来实现大小写转换,结合循环遍历字符串中的每个字符进行转换。
以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h> // 导入字符函数库
int main()
{
char str[100];
printf("请输入字符串:");
scanf("%s", str);
for (int i = 0; str[i] != '\0'; ++i) {
if (isupper(str[i])) { // 判断是否为大写字母
str[i] = tolower(str[i]); // 转换为小写字母
} else if (islower(str[i])) { // 判断是否为小写字母
str[i] = toupper(str[i]); // 转换为大写字母
}
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
这段代码中,先定义了一个字符数组`str`用于存储输入的字符串。然后使用`scanf()`函数从标准输入中读取字符串。接着使用循环遍历字符串中的每个字符,判断该字符是否为大写字母或小写字母,并使用`tolower()`和`toupper()`函数进行转换。最后输出转换后的字符串。
c语言 输入一个字符串,将大写字母转换成小写字母,并输出
好的,这是一个编程问题,我可以回答。下面是 C 语言的示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i;
printf("请输入一个字符串:");
gets(str);
for(i = 0; str[i]; i++) {
str[i] = tolower(str[i]);
}
printf("转换后的字符串:%s", str);
return 0;
}
```
该程序会让用户输入一个字符串,然后将其中的大写字母转换成小写字母,并输出结果。注意使用 `tolower` 函数将字母转换成小写。
相关推荐
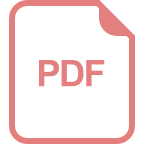
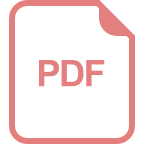












