优化这段代码: @Override public void exportExcel120(CertfInfoOrderQueryVo vo, HttpServletResponse response) { try { // 根据id查询数据 Page<DasymesCertfInfoPojo> page = new Page<>(1, 10); IPage<DasymesCertfInfoPojo> iPage = infoMapper.openQuery(page, vo); List<DasymesCertfInfoPojo> dataList = iPage.getRecords(); if (dataList == null || dataList.size() == 0) return; // 获取下载模板 InputStream template = DasymesQltyMpCertfInfoModServiceImpl.class.getClassLoader().getResourceAsStream("交付120厂产品合格证.xlsx"); if (template == null) return; DasymesCertfInfoPojo info = dataList.get(0); // 获取静态数据 Map<String, String> staticSource = getStaticSource(info); // 获取动态数据 List<DynamicSource> dynamicSourceList = getDynamicSourceList(info.getAttachedList()); Map<ByteArrayOutputStream, XSSFClientAnchor> imgMap = new HashMap<>(); ByteArrayOutputStream userByteArrayOut = new ByteArrayOutputStream(); File file = new File("D:\hegezhengtupian\"+ UserCache.getThreadLocalUser().getUserRealName() +".png"); if (file.canRead()) { bufferImg = ImageIO.read(file); ImageIO.write(bufferImg, "png", userByteArrayOut); XSSFClientAnchor userAnchor = new XSSFClientAnchor(0, 0, 0, 0, 1, 13, 2, 14); userAnchor.setAnchorType(ClientAnchor.AnchorType.MOVE_AND_RESIZE); imgMap.put(userByteArrayOut, userAnchor); } Workbook workbook = ExcelTemplateUtil.buildByTemplate(template, staticSource, dynamicSourceList, imgMap); ExcelTemplateUtil.save(workbook, "交付120厂产品合格证", response); // ExcelTemplateUtil.save(workbook, "D:\工作\文档\template.xlsx"); } catch (Exception e) { throw new RuntimeException("导出失败,请联系管理员"); } } // 编辑动态数据 private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) { List<Map<String, String>> mapList = new ArrayList<>(); if (itemModList == null || itemModList.size() == 0) { Map<String, String> map = new HashMap<>(); map.put("no", null); map.put("name", null); map.put("portion", null); mapList.add(map); return DynamicSource.createList("detail", mapList); } for (int i = 1; i <= itemModList.size(); i++) { DasymesQltyMpCertfInfoItemMod item = itemModList.get(i - 1); Map<String, String> map = new HashMap<>(); map.put("no", i + ""); map.put("name", item.getItemName()); map.put("portion", item.getPortion()); mapList.add(map); } return DynamicSource.createList("detail", mapList); }
时间: 2024-03-02 08:50:20 浏览: 21
1. 将方法拆分成更小的方法,提高可读性和可维护性。
2. 使用 try-with-resources 语句来自动关闭资源。
3. 对于文件路径,使用 File.separator 替代硬编码的路径分隔符。
4. 避免使用 magic number ,使用常量或枚举类型来代替。
5. 在使用集合时,使用 isEmpty() 方法代替 size() == 0。
6. 使用 stream() 方法来代替 for 循环。
7. 使用 lambda 表达式来代替匿名内部类。
8. 在捕获异常时,应该尽可能地捕获特定的异常。
优化后的代码如下:
```java
@Override
public void exportExcel120(CertfInfoOrderQueryVo vo, HttpServletResponse response) {
try (InputStream template = DasymesQltyMpCertfInfoModServiceImpl.class.getClassLoader().getResourceAsStream("交付120厂产品合格证.xlsx")) {
Page<DasymesCertfInfoPojo> page = new Page<>(1, PAGE_SIZE);
IPage<DasymesCertfInfoPojo> iPage = infoMapper.openQuery(page, vo);
List<DasymesCertfInfoPojo> dataList = iPage.getRecords();
if (dataList.isEmpty()) {
return;
}
DasymesCertfInfoPojo info = dataList.get(0);
Map<String, String> staticSource = getStaticSource(info);
List<DynamicSource> dynamicSourceList = getDynamicSourceList(info.getAttachedList());
Map<ByteArrayOutputStream, XSSFClientAnchor> imgMap = getImageMap();
Workbook workbook = ExcelTemplateUtil.buildByTemplate(template, staticSource, dynamicSourceList, imgMap);
ExcelTemplateUtil.save(workbook, "交付120厂产品合格证", response);
} catch (IOException e) {
throw new RuntimeException("导出失败,请联系管理员");
}
}
private Map<String, String> getStaticSource(DasymesCertfInfoPojo info) {
Map<String, String> staticSource = new HashMap<>();
staticSource.put("certfNo", info.getCertfNo());
staticSource.put("prodName", info.getProdName());
staticSource.put("spec", info.getSpec());
staticSource.put("prodNo", info.getProdNo());
staticSource.put("prodDate", info.getProdDate());
staticSource.put("expDate", info.getExpDate());
staticSource.put("prodUnit", info.getProdUnit());
staticSource.put("prodAddr", info.getProdAddr());
staticSource.put("qualityGrade", info.getQualityGrade());
staticSource.put("packing", info.getPacking());
staticSource.put("remark", info.getRemark());
return staticSource;
}
private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) {
List<Map<String, String>> mapList = new ArrayList<>();
if (itemModList.isEmpty()) {
Map<String, String> map = new HashMap<>();
map.put("no", null);
map.put("name", null);
map.put("portion", null);
mapList.add(map);
return DynamicSource.createList("detail", mapList);
}
return itemModList.stream()
.map(item -> {
Map<String, String> map = new HashMap<>();
map.put("no", String.valueOf(itemModList.indexOf(item) + 1));
map.put("name", item.getItemName());
map.put("portion", item.getPortion());
return map;
})
.collect(Collectors.collectingAndThen(Collectors.toList(), DynamicSource::createList));
}
private Map<ByteArrayOutputStream, XSSFClientAnchor> getImageMap() throws IOException {
Map<ByteArrayOutputStream, XSSFClientAnchor> imgMap = new HashMap<>();
ByteArrayOutputStream userByteArrayOut = new ByteArrayOutputStream();
File file = new File("D:" + File.separator + "hegezhengtupian" + File.separator + UserCache.getThreadLocalUser().getUserRealName() + ".png");
if (file.canRead()) {
BufferedImage bufferImg = ImageIO.read(file);
ImageIO.write(bufferImg, "png", userByteArrayOut);
XSSFClientAnchor userAnchor = new XSSFClientAnchor(0, 0, 0, 0, 1, 13, 2, 14);
userAnchor.setAnchorType(ClientAnchor.AnchorType.MOVE_AND_RESIZE);
imgMap.put(userByteArrayOut, userAnchor);
}
return imgMap;
}
```
相关推荐
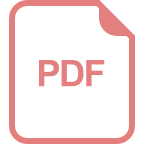
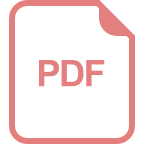















