1. 建立单链表,并在单链表上实现插入、删除和查找操作
时间: 2024-05-16 18:18:07 浏览: 82
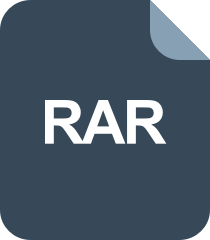
单链表的建立、插入、删除、查找.rar_单链表的建立、插入、删除、查找_插入删除

好的,我可以帮您实现这个要求。首先,我们来定义单链表的数据结构:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
```
其中,val 表示节点的值,next 表示下一个节点的指针。
接下来,我们来实现插入、删除和查找操作。
1. 插入操作
插入操作需要指定插入位置和插入的值。如果要在链表的头部插入节点,我们只需要将新节点的 next 指针指向链表的头节点即可;如果要在链表的中间或者尾部插入节点,则需要先找到插入位置,然后将新节点的 next 指针指向插入位置的下一个节点,最后将插入位置的 next 指针指向新节点。
```python
def insert_node(head, pos, val):
new_node = ListNode(val)
if pos == 0:
new_node.next = head
head = new_node
else:
cur = head
for i in range(pos-1):
cur = cur.next
new_node.next = cur.next
cur.next = new_node
return head
```
2. 删除操作
删除操作需要指定要删除的节点的位置。如果要删除链表的头节点,我们只需要将头节点的 next 指针指向头节点的下一个节点即可;如果要删除链表的中间或者尾部节点,则需要先找到要删除节点的前一个节点,然后将前一个节点的 next 指针指向要删除节点的下一个节点。
```python
def delete_node(head, pos):
if pos == 0:
head = head.next
else:
cur = head
for i in range(pos-1):
cur = cur.next
cur.next = cur.next.next
return head
```
3. 查找操作
查找操作需要指定要查找的节点的值。我们可以从链表的头节点开始遍历,逐个比较节点的值,直到找到目标节点或者遍历到链表的末尾。
```python
def search_node(head, val):
cur = head
pos = 0
while cur:
if cur.val == val:
return pos
cur = cur.next
pos += 1
return -1
```
完整代码如下:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def insert_node(head, pos, val):
new_node = ListNode(val)
if pos == 0:
new_node.next = head
head = new_node
else:
cur = head
for i in range(pos-1):
cur = cur.next
new_node.next = cur.next
cur.next = new_node
return head
def delete_node(head, pos):
if pos == 0:
head = head.next
else:
cur = head
for i in range(pos-1):
cur = cur.next
cur.next = cur.next.next
return head
def search_node(head, val):
cur = head
pos = 0
while cur:
if cur.val == val:
return pos
cur = cur.next
pos += 1
return -1
```
使用示例:
```python
# 创建链表 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))
# 在链表的第 2 个位置插入节点 6
head = insert_node(head, 1, 6)
# 链表变为 1 -> 6 -> 2 -> 3 -> 4 -> 5
# 删除链表的第 3 个节点
head = delete_node(head, 2)
# 链表变为 1 -> 6 -> 3 -> 4 -> 5
# 查找值为 3 的节点的位置
pos = search_node(head, 3)
print(pos) # 输出 2
```
阅读全文
相关推荐
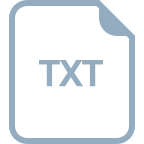
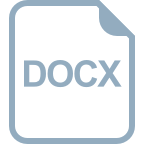
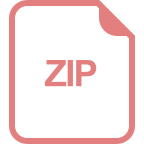
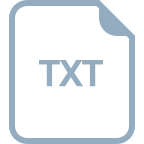
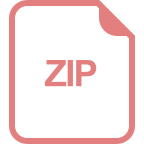
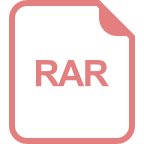
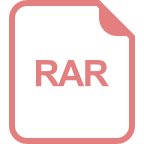
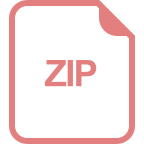
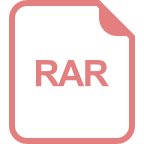
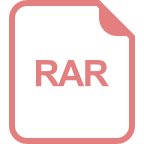
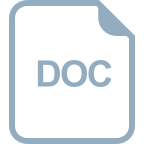
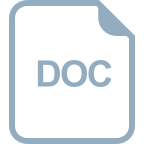