完成一个密码锁工程
时间: 2023-12-03 10:46:18 浏览: 87
好的,我可以指导你完成一个简单的密码锁工程。首先,你需要准备以下材料:
- Arduino开发板
- 4x4矩阵键盘模块
- 16x2字符液晶显示屏模块
- 杜邦线若干
- 电位器
- 电子面包板
- 9V电池和电池座(或者电源适配器)
接下来,我们按照以下步骤来实现密码锁:
1. 连接矩阵键盘模块
将矩阵键盘模块的VCC,GND分别连接到Arduino的5V,GND引脚。然后将矩阵键盘模块的行列引脚连接到Arduino的数字引脚,具体连接方式可以查看该模块的说明书。
2. 连接16x2字符液晶显示屏模块
将16x2字符液晶显示屏模块的VCC,GND分别连接到Arduino的5V,GND引脚。然后将显示屏模块的RS,E,D4,D5,D6,D7引脚连接到Arduino的数字引脚,具体连接方式可以查看该模块的说明书。
3. 连接电位器
将电位器的中间引脚连接到Arduino的模拟引脚A0,两侧引脚分别连接到5V和GND。
4. 编写程序
在Arduino IDE中编写程序,具体实现步骤如下:
(1) 定义矩阵键盘模块的行列引脚:
```c
const byte ROWS = 4;
const byte COLS = 4;
byte rowPins[ROWS] = { 9, 8, 7, 6 };
byte colPins[COLS] = { 5, 4, 3, 2 };
```
(2) 定义密码,初始化密码为"1234":
```c
char password[] = "1234";
```
(3) 定义变量,存储用户输入的密码:
```c
char input[5];
int count = 0;
```
(4) 定义LCD显示屏对象:
```c
LiquidCrystal lcd(12, 11, 10, 9, 8, 7, 6);
```
(5) 初始化LCD显示屏:
```c
lcd.begin(16, 2);
```
(6) 实现密码输入:
```c
void getInput() {
char key = keypad.getKey();
if(key) {
input[count] = key;
count++;
lcd.print("*");
delay(100);
}
}
```
(7) 验证密码:
```c
void checkPassword() {
if(count == 4) {
if(strcmp(password, input) == 0) {
lcd.clear();
lcd.print("Password correct!");
delay(2000);
lcd.clear();
count = 0;
memset(input, 0, sizeof(input));
} else {
lcd.clear();
lcd.print("Password incorrect!");
delay(2000);
lcd.clear();
count = 0;
memset(input, 0, sizeof(input));
}
}
}
```
(8) 实现电位器控制亮度:
```c
void setBacklight() {
int value = analogRead(A0);
int brightness = map(value, 0, 1023, 0, 255);
lcd.setBacklight(brightness);
}
```
(9) 完整程序代码:
```c
#include <Keypad.h>
#include <LiquidCrystal.h>
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = { 9, 8, 7, 6 };
byte colPins[COLS] = { 5, 4, 3, 2 };
char password[] = "1234";
char input[5];
int count = 0;
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS);
LiquidCrystal lcd(12, 11, 10, 9, 8, 7, 6);
void setup() {
lcd.begin(16, 2);
}
void loop() {
getInput();
checkPassword();
setBacklight();
}
void getInput() {
char key = keypad.getKey();
if(key) {
input[count] = key;
count++;
lcd.print("*");
delay(100);
}
}
void checkPassword() {
if(count == 4) {
if(strcmp(password, input) == 0) {
lcd.clear();
lcd.print("Password correct!");
delay(2000);
lcd.clear();
count = 0;
memset(input, 0, sizeof(input));
} else {
lcd.clear();
lcd.print("Password incorrect!");
delay(2000);
lcd.clear();
count = 0;
memset(input, 0, sizeof(input));
}
}
}
void setBacklight() {
int value = analogRead(A0);
int brightness = map(value, 0, 1023, 0, 255);
lcd.setBacklight(brightness);
}
```
5. 测试
将Arduino开发板连接到电脑,并上传程序。然后按照密码锁设置的密码进行测试,输入正确密码,显示"Password correct!",输入错误密码,显示"Password incorrect!",亮度可以通过电位器进行调节。
以上就是一个简单的密码锁工程实现流程。
阅读全文
相关推荐
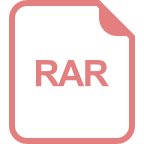
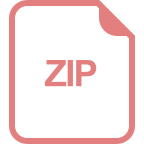
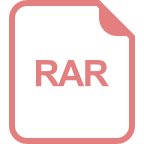
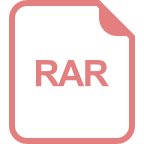
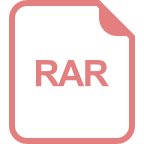
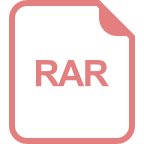
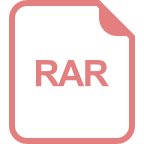
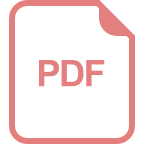
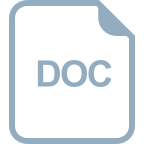
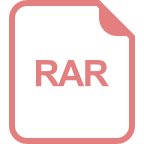
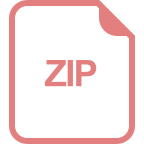
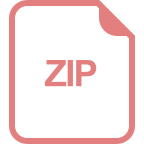
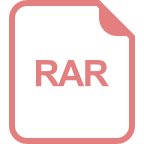
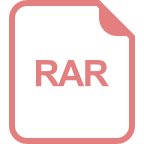
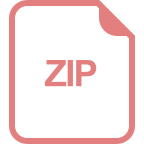
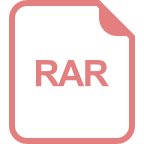
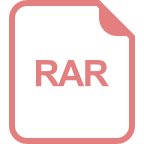