Python执行删除sql语句
时间: 2023-07-06 22:35:02 浏览: 131
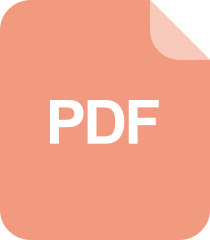
MySQL DELETE 语句
可以使用Python的mysql.connector模块来执行删除SQL语句,具体步骤如下:
1. 安装mysql.connector模块:可以使用pip命令安装,如下所示:
```
pip install mysql-connector-python
```
2. 导入模块:在Python脚本中,使用以下代码导入mysql.connector模块:
```
import mysql.connector
```
3. 连接到MySQL数据库:使用以下代码连接到MySQL数据库:
```
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
```
其中,"localhost"为MySQL服务器地址,"yourusername"为MySQL用户名,"yourpassword"为MySQL密码,"yourdatabase"为要连接的数据库名称。
4. 创建游标对象:使用以下代码创建游标对象:
```
mycursor = mydb.cursor()
```
5. 执行SQL语句:使用以下代码执行删除SQL语句:
```
sql = "DELETE FROM yourtable WHERE yourcondition"
mycursor.execute(sql)
mydb.commit()
```
其中,"yourtable"为要删除数据的表名称,"yourcondition"为删除数据的条件。
6. 关闭数据库连接:使用以下代码关闭数据库连接:
```
mydb.close()
```
完整的Python删除SQL语句的示例代码如下所示:
```
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
mycursor = mydb.cursor()
sql = "DELETE FROM yourtable WHERE yourcondition"
mycursor.execute(sql)
mydb.commit()
mydb.close()
```
阅读全文
相关推荐
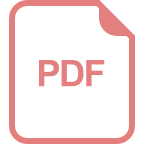
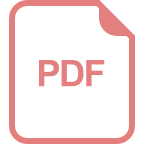
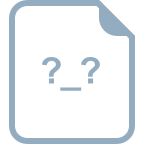
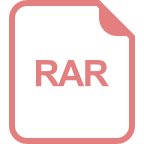
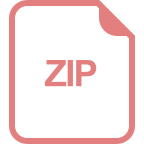
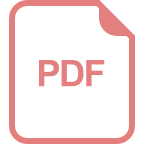
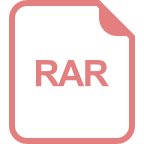
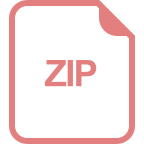
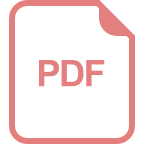
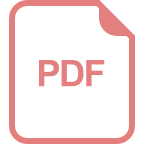
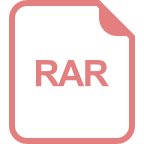
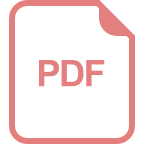
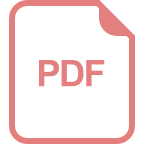
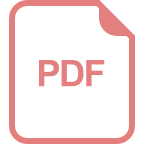
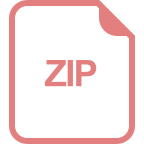
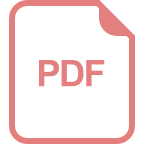
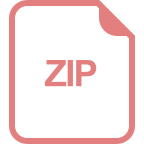
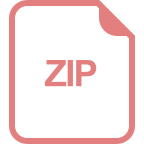