用Django写一个云笔记项目:项目有两个功能模块,用户模块 功能:用户注册、用户登录 字段:用户名、密码,云笔记模块 功能:展示首页、对笔记进行增删改查 字段:标题、内容、新增时间、修改时间 界面: 首页要求未登录状态显示登录和注册按钮,已登录状态显示欢迎用户以及查看笔记按钮; 笔记列表界面要求用表格展示所有笔记,并且每个笔记包含修改和删除按钮,界面顶部显示欢迎用户以及新增笔记按钮; 新增和修改界面要求包含标题、内容以及提交按钮 项目里所有的数据用静态字典实现
时间: 2023-05-27 08:02:07 浏览: 252
,不需要用数据库。
项目结构如下:
```
note/
├── __init__.py
├── settings.py
├── urls.py
└── views.py
templates/
├── base.html
├── home.html
├── login.html
├── note_list.html
├── note_form.html
static/
└── css/
└── style.css
```
1. 首先,创建Django项目和应用:
```
django-admin startproject note_project
cd note_project
python manage.py startapp note
```
2. 在`note`应用下,创建两个视图函数:
```python
# note/views.py
from django.shortcuts import render, redirect
# 用户注册视图函数
def register(request):
if request.method == 'POST':
# 处理用户提交的表单数据
return redirect('login')
return render(request, 'register.html')
# 用户登录视图函数
def login(request):
if request.method == 'POST':
# 处理用户提交的表单数据
return redirect('home')
return render(request, 'login.html')
# 首页视图函数
def home(request):
user = None # 假设用户还未登录
if user:
return render(request, 'home.html', {'username': user})
else:
return render(request, 'home.html')
# 笔记列表视图函数
def note_list(request):
notes = [] # 假设笔记为空列表
return render(request, 'note_list.html', {'notes': notes})
# 新增和修改笔记视图函数
def note_form(request):
return render(request, 'note_form.html')
```
3. 创建对应的视图模板:
```html
<!-- templates/login.html -->
{% extends 'base.html' %}
{% block content %}
<h1>Login</h1>
<form method="post">
{% csrf_token %}
<!-- 表单字段 -->
<button type="submit">Submit</button>
</form>
{% endblock %}
<!-- templates/register.html -->
{% extends 'base.html' %}
{% block content %}
<h1>Register</h1>
<form method="post">
{% csrf_token %}
<!-- 表单字段 -->
<button type="submit">Submit</button>
</form>
{% endblock %}
<!-- templates/home.html -->
{% extends 'base.html' %}
{% block content %}
{% if username %}
<p>Welcome, {{ username }}!</p>
<a href="{% url 'note_list' %}" class="button">View Notes</a>
{% else %}
<p>Please log in or register to continue.</p>
<a href="{% url 'login' %}" class="button">Login</a>
<a href="{% url 'register' %}" class="button">Register</a>
{% endif %}
{% endblock %}
<!-- templates/note_list.html -->
{% extends 'base.html' %}
{% block content %}
<h1>Note List</h1>
<a href="{% url 'note_form' %}" class="button">Add Note</a>
<table>
<thead>
<tr>
<th>Title</th>
<th>Content</th>
<th>Created At</th>
<th>Updated At</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for note in notes %}
<tr>
<td>{{ note.title }}</td>
<td>{{ note.content }}</td>
<td>{{ note.created_at }}</td>
<td>{{ note.updated_at }}</td>
<td>
<a href="{% url 'note_form' %}?id={{ note.id }}" class="button">Edit</a>
<a href="#">Delete</a>
</td>
</tr>
{% empty %}
<tr>
<td colspan="5">No notes found.</td>
</tr>
{% endfor %}
</tbody>
</table>
{% endblock %}
<!-- templates/note_form.html -->
{% extends 'base.html' %}
{% block content %}
<h1>{% if id %}Edit Note{% else %}Add Note{% endif %}</h1>
<form method="post">
{% csrf_token %}
<label for="title">Title</label>
<input type="text" name="title" id="title" value="{{ note.title }}">
<label for="content">Content</label>
<textarea name="content" id="content">{{ note.content }}</textarea>
<button type="submit">{% if id %}Save{% else %}Add{% endif %}</button>
</form>
{% endblock %}
<!-- templates/base.html -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>{% block title %}{% endblock %}</title>
<link rel="stylesheet" type="text/css" href="{% static 'css/style.css' %}">
</head>
<body>
<div class="container">
{% block content %}
{% endblock %}
</div>
</body>
</html>
```
4. 在`note_project/settings.py`中添加应用和模板引擎配置:
```python
# note_project/settings.py
INSTALLED_APPS = [
'note',
]
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [
BASE_DIR / 'templates',
],
'APP_DIRS': True,
'OPTIONS': {},
},
]
```
5. 在`note_project/urls.py`中添加路由映射:
```python
# note_project/urls.py
from django.urls import path
from note.views import register, login, home, note_list, note_form
urlpatterns = [
path('register/', register, name='register'),
path('login/', login, name='login'),
path('home/', home, name='home'),
path('notes/', note_list, name='note_list'),
path('notes/form/', note_form, name='note_form'),
]
```
6. 运行Django应用并访问相应的页面:
```
python manage.py runserver
```
7. 实现数据操作:
我们可以定义一些静态的数据来模拟笔记数据。在`views.py`中添加以下代码:
```python
# note/views.py
from datetime import datetime
...
# 定义一个静态的笔记数据列表
notes_data = [
{'id': 1, 'title': 'Note 1', 'content': 'This is note 1.', 'created_at': datetime.now(), 'updated_at': datetime.now()},
{'id': 2, 'title': 'Note 2', 'content': 'This is note 2.', 'created_at': datetime.now(), 'updated_at': datetime.now()},
{'id': 3, 'title': 'Note 3', 'content': 'This is note 3.', 'created_at': datetime.now(), 'updated_at': datetime.now()},
]
def note_list(request):
notes = notes_data # 使用静态的笔记数据列表
return render(request, 'note_list.html', {'notes': notes})
def note_form(request):
if request.GET.get('id'):
# 根据笔记id获取笔记对象
note = [n for n in notes_data if n['id'] == int(request.GET.get('id'))][0]
else:
note = {'id': None, 'title': '', 'content': '', 'created_at': None, 'updated_at': None}
if request.method == 'POST':
# 处理表单提交数据
note['title'] = request.POST.get('title')
note['content'] = request.POST.get('content')
note['updated_at'] = datetime.now()
if note['id']:
# 更新已有笔记
idx = notes_data.index(note)
notes_data[idx] = note
else:
# 添加新笔记
note['id'] = len(notes_data) + 1
note['created_at'] = datetime.now()
notes_data.append(note)
return redirect('note_list')
return render(request, 'note_form.html', {'note': note})
```
这里使用了一个静态的笔记数据列表,当在笔记列表界面点击编辑按钮时,会通过笔记id获取相应的笔记对象,并在笔记表单页面给出该笔记的标题和内容。在笔记表单页面使用相同的表单来处理笔记的新增和编辑操作,根据`note['id']`的值判断对笔记进行何种操作。在数据操作完成后,跳转到笔记列表页面。
现在我们已经实现了一个简单的云笔记Django项目,运行Django应用并在浏览器中访问相应的URL可以测试该项目的各项功能。
阅读全文
相关推荐
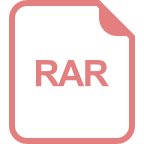




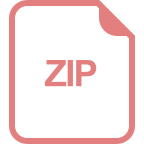
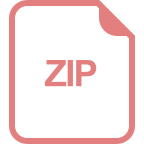
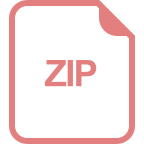
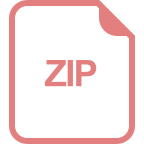
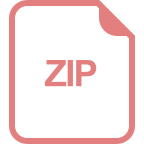
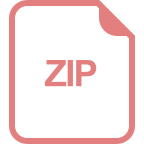
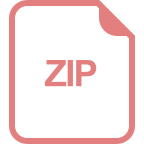
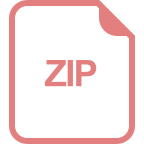
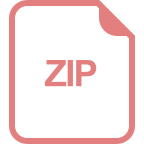
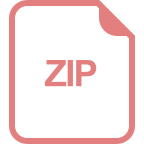
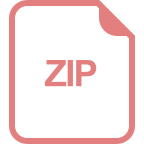
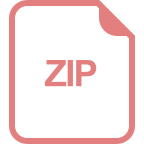