写个树莓派下通过gpio获取中断的列子
时间: 2023-08-10 15:09:08 浏览: 51
好的,下面是一个树莓派下通过GPIO获取中断的示例代码:
```python
import RPi.GPIO as GPIO
# 初始化GPIO
GPIO.setmode(GPIO.BCM)
# 设置GPIO
GPIO.setup(17, GPIO.IN, pull_up_down=GPIO.PUD_UP)
# 定义中断回调函数
def my_callback(channel):
print("Button was pressed!")
# 监听GPIO
GPIO.add_event_detect(17, GPIO.FALLING, callback=my_callback, bouncetime=200)
# 主循环
try:
while True:
pass
except KeyboardInterrupt:
GPIO.cleanup()
```
这个示例代码监听GPIO 17的下降沿,当检测到下降沿时,会调用`my_callback`回调函数进行处理。注意,这个例子中使用了`GPIO.PUD_UP`来开启上拉电阻,以确保GPIO在未连接时保持高电平状态。另外,`bouncetime`参数可以用来消除机械按键的抖动问题。当然,这只是一个简单的示例,实际应用中需要根据具体情况进行调整。
相关问题
写个C语言树莓派下通过gpio获取中断的
你可以使用wiringPi库来实现在树莓派上通过GPIO获取中断。下面是一个示例代码:
```c
#include <stdio.h>
#include <wiringPi.h>
#define BUTTON_PIN 17
void buttonInterrupt(void) {
printf("Button pressed!\n");
}
int main(void) {
if (wiringPiSetupGpio() == -1) {
printf("wiringPi setup failed\n");
return 1;
}
pinMode(BUTTON_PIN, INPUT);
pullUpDnControl(BUTTON_PIN, PUD_UP);
wiringPiISR(BUTTON_PIN, INT_EDGE_FALLING, &buttonInterrupt);
while (1) {
// do something else
}
return 0;
}
```
在这个示例中,我们使用GPIO17作为输入,当它被按下时,会触发中断,并调用`buttonInterrupt`函数。在`main`函数中,我们使用`wiringPiISR`函数来注册中断,并使用`INT_EDGE_FALLING`指定当输入信号从高电平变为低电平时触发中断。最后,在程序的主循环中,我们可以做其他的事情,而不是一直等待中断的发生。
写一个PIC32MZ的GPIO中断例子
当然可以!以下是一个基于PIC32MZ的GPIO中断的例子:
```c
#include <xc.h>
// 定义中断处理函数
void __ISR(_EXTERNAL_0_VECTOR, IPL1AUTO) ExternalInterruptHandler(void)
{
// 处理中断事件
// 在这里添加你的代码
// 例如,可以改变某个GPIO引脚的状态或执行其他操作
// ...
// 清除中断标志位
IFS0CLR = _IFS0_INT0IF_MASK;
}
int main(void)
{
// 初始化GPIO引脚和外部中断
// 设置GPIO引脚为输入模式
TRISGSET = _TRISG_TRISG6_MASK; // 将RG6设置为输入
// 配置外部中断触发边沿
INTCONbits.INT0EP = 0; // 触发上升沿(或下降沿,根据需要设置)
// 配置中断优先级和使能
IPC0bits.INT0IP = 1; // 设置中断优先级为1(根据需要设置)
IEC0SET = _IEC0_INT0IE_MASK; // 使能INT0外部中断
// 启用全局中断
asm volatile("ei");
while (1)
{
// 在这里可以执行其他任务
// ...
}
return 0;
}
```
这个例子演示了如何在PIC32MZ上使用外部中断。在主函数中,我们首先配置了一个GPIO引脚(这里以RG6为例)作为输入,并设置了外部中断的触发边沿(上升沿或下降沿)。然后,我们配置了中断优先级和使能,并启用全局中断。在中断处理函数中,你可以添加代码来处理中断事件,例如改变其他GPIO引脚的状态或执行其他操作。
请注意,具体的配置可能会因你使用的PIC32MZ型号和开发环境而有所不同。请参考相关文档和手册以获取更详细的信息。
相关推荐
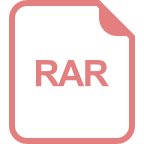












