android实现一个动态实现新闻阅读功能的代码和布局文件
时间: 2024-03-13 07:43:56 浏览: 15
以下是一个简单的动态实现新闻阅读功能的 Android 代码和布局文件示例。这个示例使用了 RecyclerView 和 CardView 来显示新闻列表和新闻详情。
布局文件 news_list_item.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="8dp"
app:cardCornerRadius="8dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ImageView
android:id="@+id/news_image"
android:layout_width="match_parent"
android:layout_height="200dp"
android:scaleType="centerCrop" />
<TextView
android:id="@+id/news_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="8dp"
android:textSize="18sp"
android:textColor="@android:color/black"
android:textStyle="bold" />
<TextView
android:id="@+id/news_date"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginEnd="8dp"
android:textSize="14sp"
android:textColor="@android:color/darker_gray" />
</LinearLayout>
</androidx.cardview.widget.CardView>
```
布局文件 news_detail.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<ImageView
android:id="@+id/news_detail_image"
android:layout_width="match_parent"
android:layout_height="200dp"
android:scaleType="centerCrop" />
<TextView
android:id="@+id/news_detail_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:textSize="24sp"
android:textColor="@android:color/black"
android:textStyle="bold" />
<TextView
android:id="@+id/news_detail_date"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:textSize="18sp"
android:textColor="@android:color/darker_gray" />
<TextView
android:id="@+id/news_detail_content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:textSize="18sp"
android:textColor="@android:color/black" />
</LinearLayout>
</ScrollView>
```
Java 代码:
```java
public class NewsListAdapter extends RecyclerView.Adapter<NewsListAdapter.ViewHolder> {
private List<News> mNewsList;
private Context mContext;
public NewsListAdapter(Context context, List<News> newsList) {
mContext = context;
mNewsList = newsList;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(mContext).inflate(R.layout.news_list_item, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
final News news = mNewsList.get(position);
holder.mNewsTitle.setText(news.getTitle());
holder.mNewsDate.setText(news.getDate());
Glide.with(mContext).load(news.getImageUrl()).into(holder.mNewsImage);
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(mContext, NewsDetailActivity.class);
intent.putExtra("news", news);
mContext.startActivity(intent);
}
});
}
@Override
public int getItemCount() {
return mNewsList.size();
}
static class ViewHolder extends RecyclerView.ViewHolder {
ImageView mNewsImage;
TextView mNewsTitle;
TextView mNewsDate;
ViewHolder(@NonNull View itemView) {
super(itemView);
mNewsImage = itemView.findViewById(R.id.news_image);
mNewsTitle = itemView.findViewById(R.id.news_title);
mNewsDate = itemView.findViewById(R.id.news_date);
}
}
}
public class NewsDetailActivity extends AppCompatActivity {
private ImageView mNewsDetailImage;
private TextView mNewsDetailTitle;
private TextView mNewsDetailDate;
private TextView mNewsDetailContent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.news_detail);
mNewsDetailImage = findViewById(R.id.news_detail_image);
mNewsDetailTitle = findViewById(R.id.news_detail_title);
mNewsDetailDate = findViewById(R.id.news_detail_date);
mNewsDetailContent = findViewById(R.id.news_detail_content);
Intent intent = getIntent();
News news = (News) intent.getSerializableExtra("news");
mNewsDetailTitle.setText(news.getTitle());
mNewsDetailDate.setText(news.getDate());
mNewsDetailContent.setText(news.getContent());
Glide.with(this).load(news.getImageUrl()).into(mNewsDetailImage);
}
}
```
以上代码仅供参考,具体实现方式还需要根据具体业务需求进行修改。
相关推荐
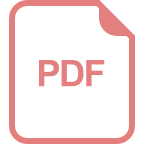
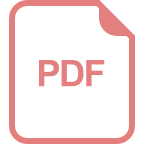
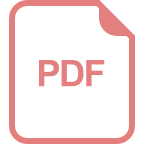














