spring-boot 实现 API 接口合并
时间: 2023-12-06 11:02:35 浏览: 34
实现 API 接口合并可以通过 Spring Boot 中的 Feign 和 Ribbon 组件来实现。具体步骤如下:
1. 在 pom.xml 中添加依赖:
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-ribbon</artifactId>
</dependency>
```
2. 创建一个 Feign 接口,用于调用需要合并的 API 接口:
```
@FeignClient(name = "service-name")
public interface ApiService {
@GetMapping("/api/path")
String getData();
}
```
3. 在 Spring Boot 启动类中添加 @EnableFeignClients 和 @RibbonClients 注解:
```
@SpringBootApplication
@EnableFeignClients
@RibbonClients(defaultConfiguration = RibbonConfiguration.class)
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
4. 创建一个 Ribbon 配置类,用于配置负载均衡策略:
```
@Configuration
public class RibbonConfiguration {
@Bean
public IRule ribbonRule() {
// 配置负载均衡策略
return new RandomRule();
}
}
```
5. 创建一个自定义的 API 接口,用于合并多个 API 接口:
```
@RestController
@RequestMapping("/api")
public class CombinedApiController {
@Autowired
private ApiService apiService;
@GetMapping("/combined")
public List<String> getDataFromMultipleApis() {
List<String> result = new ArrayList<>();
result.add(apiService.getData());
// 调用其他需要合并的接口并将结果加入到 result 中
return result;
}
}
```
通过以上步骤,就可以实现 API 接口的合并。在 CombinedApiController 中,通过调用 ApiService 中的接口来获取数据,然后将多个接口返回的数据合并在一起返回给客户端。同时,通过 Ribbon 配置负载均衡策略,可以实现对多个 API 服务的负载均衡。
相关推荐
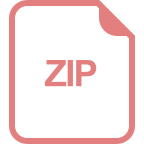
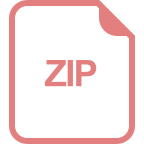
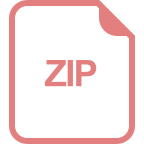














