vue3用uniapp写一个折线图
时间: 2023-07-18 20:41:39 浏览: 55
好的,下面是一个示例代码,可以用于在uniapp中绘制折线图:
```vue
<template>
<view>
<canvas canvas-id="myCanvas" style="width: 100%; height: 300px;"></canvas>
</view>
</template>
<script>
export default {
mounted() {
this.drawLineChart();
},
methods: {
drawLineChart() {
const ctx = uni.createCanvasContext('myCanvas');
const xData = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'];
const yData = [150, 200, 300, 180, 250, 220];
// 计算 y 轴最大值和最小值
const maxVal = Math.max(...yData);
const minVal = Math.min(...yData);
// 定义画布宽度和高度
const canvasWidth = uni.upx2px(750);
const canvasHeight = uni.upx2px(300);
// 定义坐标轴的起点和终点
const xAxisStart = uni.upx2px(50);
const xAxisEnd = uni.upx2px(700);
const yAxisStart = uni.upx2px(250);
const yAxisEnd = uni.upx2px(50);
// 绘制坐标轴
ctx.beginPath();
ctx.moveTo(xAxisStart, yAxisStart);
ctx.lineTo(xAxisEnd, yAxisStart);
ctx.moveTo(xAxisStart, yAxisStart);
ctx.lineTo(xAxisStart, yAxisEnd);
ctx.stroke();
// 绘制 x 轴标签
const xStep = (xAxisEnd - xAxisStart) / (xData.length - 1);
xData.forEach((item, index) => {
ctx.fillText(item, xAxisStart + index * xStep - 10, yAxisStart + 20);
});
// 绘制 y 轴标签
const yStep = (yAxisStart - yAxisEnd) / 5;
for (let i = 0; i <= 5; i++) {
const val = minVal + (maxVal - minVal) * i / 5;
ctx.fillText(val.toFixed(0), xAxisStart - 30, yAxisStart - i * yStep + 5);
}
// 绘制折线
ctx.beginPath();
ctx.strokeStyle = '#f00';
ctx.lineWidth = 2;
yData.forEach((item, index) => {
const x = xAxisStart + index * xStep;
const y = yAxisStart - (item - minVal) / (maxVal - minVal) * (yAxisStart - yAxisEnd);
if (index === 0) {
ctx.moveTo(x, y);
} else {
ctx.lineTo(x, y);
}
});
ctx.stroke();
ctx.draw();
}
}
}
</script>
```
这段代码中,通过 `uni.createCanvasContext` 创建了一个画布上下文,然后绘制了坐标轴、折线以及标签。你可以根据需求修改代码中的数据和样式。
相关推荐
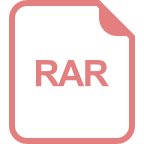
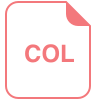
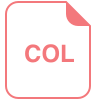
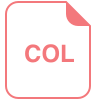
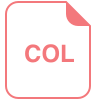
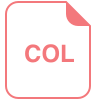









