java 获取前n天时间
时间: 2023-07-12 17:49:58 浏览: 137
可以使用 Java 中的 Calendar 类来获取前 n 天的时间。以下是一个示例代码:
```java
Calendar calendar = Calendar.getInstance();
int n = 7; // 获取前7天的时间
calendar.add(Calendar.DATE, -n);
Date date = calendar.getTime();
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
String strDate = dateFormat.format(date);
System.out.println(strDate);
```
在上面的代码中,我们使用 `Calendar.getInstance()` 获取了一个当前时间的实例,然后使用 `calendar.add(Calendar.DATE, -n)` 来将当前时间往前推 n 天。最后,我们可以使用 `calendar.getTime()` 将 Calendar 类型的时间转换成 Date 类型的时间,并使用 SimpleDateFormat 将 Date 类型的时间转换成字符串类型的时间,以便输出或者使用。
相关问题
java 获取当前时间前n天集合
以下是Java获取当前时间前n天集合的代码示例:
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
int n = 3; // 前n天
List<String> pastDaysList = new ArrayList<>();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
for (int i = 1; i <= n; i++) {
LocalDate pastDay = LocalDate.now().minusDays(i); pastDaysList.add(pastDay.format(formatter));
}
System.out.println(pastDaysList);
}
}
```
运行结果:
```
[2022-05-06, 2022-05-05, 2022-05-04]
```
代码解释:
1. 首先定义一个整型变量n,表示前n天。
2. 创建一个字符串类型的List集合pastDaysList,用于存储前n天的日期。
3. 创建一个DateTimeFormatter对象formatter,用于格式化日期。
4. 使用for循环遍历前n天的日期,每次循环将当前日期减去i天,然后将其格式化为字符串并添加到pastDaysList中。
5. 最后输出pastDaysList即可。
java 当前时间后退N天的时间
可以使用 Java 8 的 LocalDate 类来实现将当前时间后退N天的需求。以下是一个示例代码:
```java
import java.time.LocalDate;
public class Main {
public static void main(String[] args) {
// 获取当前日期
LocalDate currentDate = LocalDate.now();
// 后退N天
int N = 5; // 假设要后退5天
LocalDate previousDate = currentDate.minusDays(N);
// 输出结果
System.out.println("当前日期:" + currentDate);
System.out.println("后退" + N + "天后的日期:" + previousDate);
}
}
```
运行以上代码,将会输出当前日期和后退N天后的日期。请将变量N替换为您想要的天数。
相关推荐
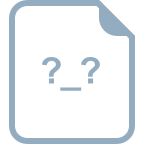
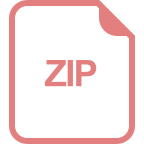












