用 Python 的 Turtle 库画一只懒羊羊
时间: 2023-11-05 14:58:07 浏览: 47
好的,让我展示一下 Python 的 Turtle 库,然后画一只懒羊羊。
首先,我们需要导入 Turtle 库:
```python
import turtle
```
然后,我们可以设置一些窗口的属性,例如背景颜色等:
```python
window = turtle.Screen()
window.bgcolor('#FFE4B5')
window.title('懒羊羊')
```
接下来,我们可以创建一个海龟画笔对象:
```python
pen = turtle.Turtle()
```
然后,我们就可以开始画了。首先,让我们画一个圆形的脸:
```python
pen.penup()
pen.goto(0, -100)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.circle(100)
pen.end_fill()
```
接下来,画眼睛:
```python
pen.penup()
pen.goto(-50, 50)
pen.pendown()
pen.begin_fill()
pen.fillcolor('white')
pen.circle(20)
pen.end_fill()
pen.penup()
pen.goto(50, 50)
pen.pendown()
pen.begin_fill()
pen.fillcolor('white')
pen.circle(20)
pen.end_fill()
pen.penup()
pen.goto(-50, 65)
pen.pendown()
pen.begin_fill()
pen.fillcolor('black')
pen.circle(10)
pen.end_fill()
pen.penup()
pen.goto(50, 65)
pen.pendown()
pen.begin_fill()
pen.fillcolor('black')
pen.circle(10)
pen.end_fill()
```
然后,画嘴巴:
```python
pen.penup()
pen.goto(-30, 10)
pen.pendown()
pen.width(5)
pen.right(45)
pen.forward(60)
pen.right(90)
pen.forward(60)
```
最后,画两只脚:
```python
pen.penup()
pen.goto(-50, -150)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.width(1)
pen.right(45)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.end_fill()
pen.penup()
pen.goto(0, -150)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.width(1)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.end_fill()
```
最终,完整的代码如下:
```python
import turtle
window = turtle.Screen()
window.bgcolor('#FFE4B5')
window.title('懒羊羊')
pen = turtle.Turtle()
pen.penup()
pen.goto(0, -100)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.circle(100)
pen.end_fill()
pen.penup()
pen.goto(-50, 50)
pen.pendown()
pen.begin_fill()
pen.fillcolor('white')
pen.circle(20)
pen.end_fill()
pen.penup()
pen.goto(50, 50)
pen.pendown()
pen.begin_fill()
pen.fillcolor('white')
pen.circle(20)
pen.end_fill()
pen.penup()
pen.goto(-50, 65)
pen.pendown()
pen.begin_fill()
pen.fillcolor('black')
pen.circle(10)
pen.end_fill()
pen.penup()
pen.goto(50, 65)
pen.pendown()
pen.begin_fill()
pen.fillcolor('black')
pen.circle(10)
pen.end_fill()
pen.penup()
pen.goto(-30, 10)
pen.pendown()
pen.width(5)
pen.right(45)
pen.forward(60)
pen.right(90)
pen.forward(60)
pen.penup()
pen.goto(-50, -150)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.width(1)
pen.right(45)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.end_fill()
pen.penup()
pen.goto(0, -150)
pen.pendown()
pen.begin_fill()
pen.fillcolor('#F5DEB3')
pen.width(1)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.end_fill()
turtle.done()
```
期望的输出应该是下面的形状:

相关推荐
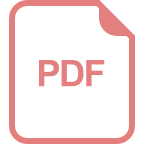
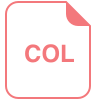













