springboot整合mybatis实现关联查询
时间: 2023-09-12 13:10:27 浏览: 150
实现关联查询需要使用MyBatis的多表查询功能,并在Spring Boot中进行整合。以下是一些基本步骤:
1. 在pom.xml中添加MyBatis和MySQL依赖。
2. 创建实体类和Mapper接口。
3. 在MyBatis的Mapper配置文件中编写关联查询语句。
4. 在Spring Boot中配置MyBatis。
5. 在Service中调用Mapper接口方法实现关联查询。
下面是一个示例:
1. 添加依赖
```
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
2. 创建实体类和Mapper接口
```
public class User {
private Long id;
private String name;
private Integer age;
private List<Article> articles;
// getter and setter
}
public class Article {
private Long id;
private String title;
private String content;
private Long userId;
// getter and setter
}
public interface UserMapper {
User getUserById(Long id);
}
```
3. 编写关联查询语句
在MyBatis的Mapper配置文件中编写关联查询语句,例如:
```
<select id="getUserById" resultType="User">
SELECT u.*, a.id as article_id, a.title as article_title, a.content as article_content
FROM user u
LEFT JOIN article a ON u.id = a.user_id
WHERE u.id = #{id}
</select>
```
4. 配置MyBatis
在application.properties文件中配置MyBatis的数据源、Mapper扫描路径等:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
mybatis.mapper-locations=classpath*:mapper/*.xml
```
5. 在Service中调用Mapper接口方法实现关联查询
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(Long id) {
return userMapper.getUserById(id);
}
}
```
以上就是使用Spring Boot整合MyBatis实现关联查询的基本步骤。
阅读全文
相关推荐
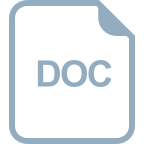
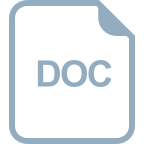
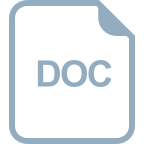

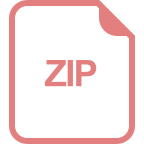
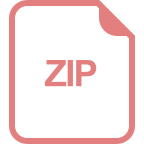
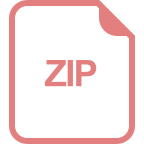
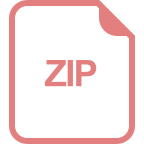
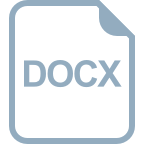
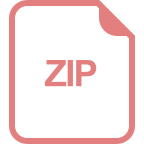
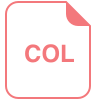
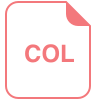

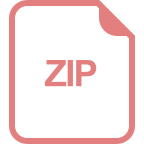
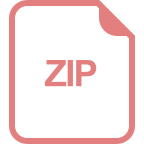
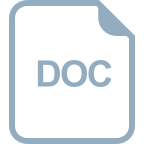
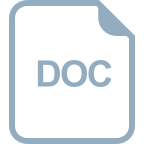
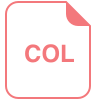
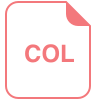