vue成绩表增删改查怎么写
时间: 2023-07-10 19:12:35 浏览: 55
Vue成绩表的增删改查可以使用Vue框架的数据绑定和方法来实现。以下是一个简单的示例代码:
HTML部分:
```
<div id="app">
<table>
<thead>
<tr>
<th>姓名</th>
<th>语文成绩</th>
<th>数学成绩</th>
<th>英语成绩</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in students">
<td>{{ item.name }}</td>
<td>{{ item.chinese }}</td>
<td>{{ item.math }}</td>
<td>{{ item.english }}</td>
<td>
<button @click="editStudent(index)">编辑</button>
<button @click="deleteStudent(index)">删除</button>
</td>
</tr>
</tbody>
</table>
<form>
<label>姓名:</label>
<input type="text" v-model="newStudent.name">
<label>语文成绩:</label>
<input type="text" v-model="newStudent.chinese">
<label>数学成绩:</label>
<input type="text" v-model="newStudent.math">
<label>英语成绩:</label>
<input type="text" v-model="newStudent.english">
<button @click.prevent="addStudent()">添加</button>
</form>
</div>
```
JS部分:
```
var app = new Vue({
el: '#app',
data: {
students: [
{ name: '张三', chinese: 80, math: 90, english: 70 },
{ name: '李四', chinese: 70, math: 80, english: 90 },
{ name: '王五', chinese: 90, math: 70, english: 80 }
],
newStudent: {
name: '',
chinese: '',
math: '',
english: ''
},
editIndex: -1
},
methods: {
addStudent: function() {
this.students.push({
name: this.newStudent.name,
chinese: this.newStudent.chinese,
math: this.newStudent.math,
english: this.newStudent.english
})
this.newStudent.name = ''
this.newStudent.chinese = ''
this.newStudent.math = ''
this.newStudent.english = ''
},
editStudent: function(index) {
this.newStudent.name = this.students[index].name
this.newStudent.chinese = this.students[index].chinese
this.newStudent.math = this.students[index].math
this.newStudent.english = this.students[index].english
this.editIndex = index
},
deleteStudent: function(index) {
this.students.splice(index, 1)
}
}
})
```
以上代码实现了一个简单的Vue成绩表增删改查功能,包括添加学生、编辑学生、删除学生等功能。其中,数据绑定使用了Vue的v-model指令,方法使用了Vue的methods属性来定义。
相关推荐
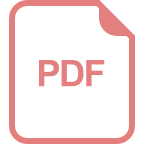














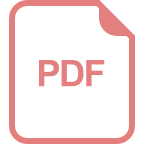