用wpf 写一个读取xml文件的程序
时间: 2023-11-20 17:06:58 浏览: 107
以下是一个使用WPF读取XML文件的示例程序:
MainWindow.xaml
```xml
<Window x:Class="WpfXmlReader.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="XML Reader" Height="350" Width="525">
<Grid>
<StackPanel>
<TextBlock Text="XML File Path:"/>
<TextBox x:Name="txtXmlFilePath"/>
<Button Content="Read XML" Click="btnReadXml_Click"/>
<ListBox x:Name="lstXmlData"/>
</StackPanel>
</Grid>
</Window>
```
MainWindow.xaml.cs
```csharp
using System.Windows;
using System.Xml;
namespace WpfXmlReader
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void btnReadXml_Click(object sender, RoutedEventArgs e)
{
lstXmlData.Items.Clear();
string xmlFilePath = txtXmlFilePath.Text.Trim();
if (!string.IsNullOrEmpty(xmlFilePath))
{
try
{
// Load the XML file
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(xmlFilePath);
// Read the XML data
XmlNodeList nodes = xmlDoc.SelectNodes("//book");
foreach (XmlNode node in nodes)
{
string title = node.SelectSingleNode("title").InnerText;
string author = node.SelectSingleNode("author").InnerText;
string price = node.SelectSingleNode("price").InnerText;
lstXmlData.Items.Add(string.Format("Title: {0}\tAuthor: {1}\tPrice: {2}", title, author, price));
}
}
catch (XmlException ex)
{
MessageBox.Show(ex.Message, "Error", MessageBoxButton.OK, MessageBoxImage.Error);
}
}
else
{
MessageBox.Show("Please enter XML file path.", "Warning", MessageBoxButton.OK, MessageBoxImage.Warning);
}
}
}
}
```
在此示例中,我们在窗口中添加了一个文本框和一个按钮,用于输入XML文件的路径并读取XML数据。我们还添加了一个列表框,用于显示读取的XML数据。
在“Read XML”按钮的单击事件处理程序中,我们首先清除列表框中的所有项。然后,我们从文本框中获取XML文件的路径,并使用XmlDocument类加载XML文件。接下来,我们选择所有的“book”节点,并使用SelectSingleNode方法读取每个节点的“title”、“author”和“price”元素的InnerText属性。最后,我们将读取的数据添加到列表框中。
如果加载XML文件时发生异常(例如,文件不存在或格式不正确),我们将通过MessageBox.Show方法显示错误消息。
阅读全文
相关推荐










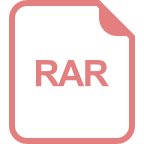







