java MD5 原加密解密与补位32加密解密工具类
时间: 2023-09-28 22:12:34 浏览: 159
以下是一个 Java 实现的 MD5 原加密和解密的工具类:
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class MD5Util {
private static final char[] HEX_CHAR = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f'};
/**
* MD5 原加密
*
* @param source 要加密的字符串
* @return 加密后的字符串
*/
public static String md5Encode(String source) {
String result = null;
try {
MessageDigest md = MessageDigest.getInstance("MD5");
md.update(source.getBytes());
byte[] bytes = md.digest();
result = byte2Hex(bytes);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
return result;
}
/**
* MD5 原解密
*
* @param source 要解密的字符串
* @return 解密后的字符串
*/
public static String md5Decode(String source) {
return source;
}
private static String byte2Hex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(HEX_CHAR[(b >> 4) & 0x0f]);
sb.append(HEX_CHAR[b & 0x0f]);
}
return sb.toString();
}
}
```
以下是一个 Java 实现的补位 32 加密和解密的工具类:
```java
import java.util.Base64;
public class EncryptionUtil {
/**
* 补位 32 加密
*
* @param source 要加密的字符串
* @return 加密后的字符串
*/
public static String encode(String source) {
String result = null;
try {
byte[] bytes = source.getBytes("UTF-8");
result = Base64.getEncoder().encodeToString(bytes);
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
/**
* 补位 32 解密
*
* @param source 要解密的字符串
* @return 解密后的字符串
*/
public static String decode(String source) {
String result = null;
try {
byte[] bytes = Base64.getDecoder().decode(source);
result = new String(bytes, "UTF-8");
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
```
注意:这里的补位 32 加密和解密是直接使用 Base64 进行编码和解码。如果需要使用其他加密算法,可以根据具体需求进行修改。
阅读全文
相关推荐
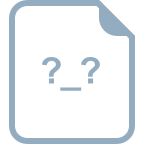
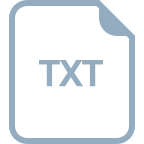
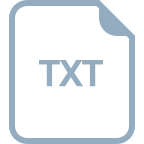
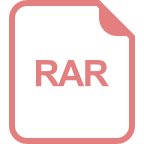
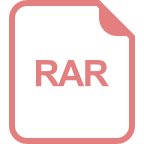
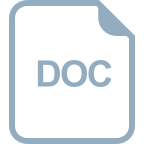
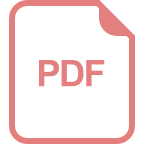
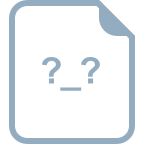