请写一个能键盘输入数据进行复数的加减乘除乘方立方运算的代码
时间: 2023-06-14 21:06:54 浏览: 128
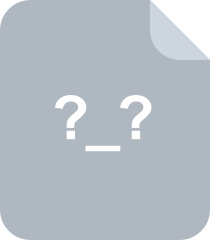
带有键盘输入的复数的复合运算java实现
以下是一个简单的 Python 代码示例,可以实现复数的加减乘除乘方立方运算:
```python
# 定义复数类
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
# 复数加法
def __add__(self, other):
return Complex(self.real + other.real, self.imag + other.imag)
# 复数减法
def __sub__(self, other):
return Complex(self.real - other.real, self.imag - other.imag)
# 复数乘法
def __mul__(self, other):
real = self.real * other.real - self.imag * other.imag
imag = self.real * other.imag + self.imag * other.real
return Complex(real, imag)
# 复数除法
def __truediv__(self, other):
denominator = other.real ** 2 + other.imag ** 2
real = (self.real * other.real + self.imag * other.imag) / denominator
imag = (self.imag * other.real - self.real * other.imag) / denominator
return Complex(real, imag)
# 复数乘方
def power(self, n):
r = (self.real ** 2 + self.imag ** 2) ** 0.5
theta = math.atan2(self.imag, self.real)
real = r ** n * math.cos(n * theta)
imag = r ** n * math.sin(n * theta)
return Complex(real, imag)
# 复数立方
def cube(self):
return self.power(3)
# 主程序
if __name__ == '__main__':
while True:
# 读取用户输入
input_str = input('请输入复数表达式(例如:2+3j):')
# 解析用户输入
try:
real, imag = map(float, input_str.split('+'))
except ValueError:
print('输入有误,请重新输入!')
continue
# 创建复数对象
c1 = Complex(real, imag)
# 读取运算符
operator = input('请输入运算符(+、-、*、/、^、3):')
# 执行运算
if operator == '+':
input_str = input('请输入另一个复数表达式(例如:2+3j):')
try:
real, imag = map(float, input_str.split('+'))
except ValueError:
print('输入有误,请重新输入!')
continue
c2 = Complex(real, imag)
result = c1 + c2
elif operator == '-':
input_str = input('请输入另一个复数表达式(例如:2+3j):')
try:
real, imag = map(float, input_str.split('+'))
except ValueError:
print('输入有误,请重新输入!')
continue
c2 = Complex(real, imag)
result = c1 - c2
elif operator == '*':
input_str = input('请输入另一个复数表达式(例如:2+3j):')
try:
real, imag = map(float, input_str.split('+'))
except ValueError:
print('输入有误,请重新输入!')
continue
c2 = Complex(real, imag)
result = c1 * c2
elif operator == '/':
input_str = input('请输入另一个复数表达式(例如:2+3j):')
try:
real, imag = map(float, input_str.split('+'))
except ValueError:
print('输入有误,请重新输入!')
continue
c2 = Complex(real, imag)
result = c1 / c2
elif operator == '^':
input_str = input('请输入乘方指数:')
try:
n = int(input_str)
except ValueError:
print('输入有误,请重新输入!')
continue
result = c1.power(n)
elif operator == '3':
result = c1.cube()
else:
print('输入有误,请重新输入!')
continue
# 输出结果
print('计算结果为:{}+{}j'.format(result.real, result.imag))
```
该程序首先定义了一个复数类 `Complex`,其中包括了复数加法、减法、乘法、除法、乘方、立方等运算的实现。
然后,程序通过一个死循环来读取用户输入,并根据用户输入的运算符执行相应的运算。当用户输入非法表达式或运算符时,程序会提示用户重新输入。最后,程序将计算结果输出到屏幕上。
请注意,由于 Python 中的虚数单位为 `j` 而不是 `i`,因此输入的复数表达式应该形如 `2+3j` 而不是 `2+3i`。
阅读全文
相关推荐
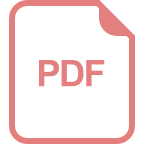
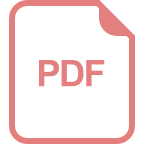
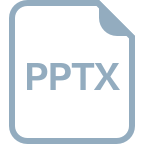
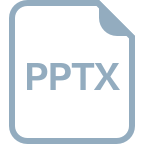
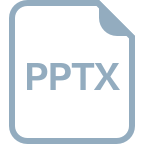
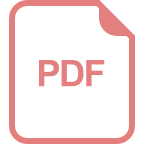
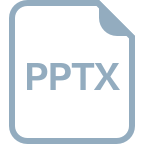
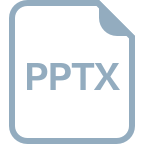
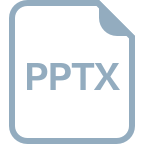
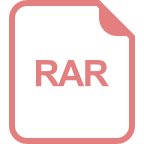
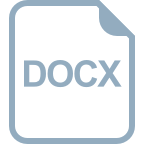
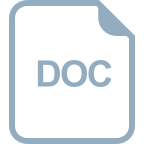