switch (image.type) { case 1: this.imagesArray.push(image.url) break; case 2: this.imagesArray.push(image.url) break; case 3: this.imagesArray.push(image.url) break; case 4: this.imagesArray.push(image.url) break; case 5: this.imagesArray.push(image.url) break; case 6: this.imagesArray.push(image.url) break; case 7: this.imagesArray.push(image.url) break; case 8: this.imagesArray.push(image.url) break; case 9: this.imagesArray.push(image.url) break; default: break; }
时间: 2024-02-14 11:23:29 浏览: 23
这是一个 JavaScript 中的 switch 语句,根据 image 对象的 type 属性的值来进行不同的操作。如果 type 的值是 1 到 9 中的任何一个,就把 image 对象的 url 属性存入 imagesArray 数组中,否则不进行任何操作。这个代码块可能是用来筛选出特定类型的图片并将它们存入数组中的。
相关问题
翻译这段代码: switch (data.code) { case 3: // need pre hot toPush('pages/home/preHot.qml', 'preHot', { makeInfo: { drinkName: getName(drink), drinkEngName: drink.engName, uuid: drink.uuid, brandUuid: drink.brandUuid, image: drink.image, textFlag: '0', params: data.data }, }) break case 2: // not make toast.show(language.makeFail, 3000) break case 1: // needn't prehot toPush('pages/home/make.qml', 'make', { forceMake: 'forceMake', drinkName: getName(drink), drinkEngName: drink.engName, uuid: drink.uuid, brandUuid: drink.brandUuid, image: drink.image, params:data.data, textFlag: '0', }) }
以下是代码的翻译:
```javascript
switch (data.code) {
case 3: // 需要预热
toPush('pages/home/preHot.qml', 'preHot', {
makeInfo: {
drinkName: getName(drink),
drinkEngName: drink.engName,
uuid: drink.uuid,
brandUuid: drink.brandUuid,
image: drink.image,
textFlag: '0',
params: data.data
},
});
break;
case 2: // 无法制作
toast.show(language.makeFail, 3000);
break;
case 1: // 不需要预热
toPush('pages/home/make.qml', 'make', {
forceMake: 'forceMake',
drinkName: getName(drink),
drinkEngName: drink.engName,
uuid: drink.uuid,
brandUuid: drink.brandUuid,
image: drink.image,
params: data.data,
textFlag: '0',
});
break;
}
```
根据 `data.code` 的不同值进行不同的操作:
- 当 `data.code` 为 3 时,需要预热。调用 `toPush` 函数,传入 `'pages/home/preHot.qml'`、`'preHot'` 和一个包含相关信息的对象。
- 当 `data.code` 为 2 时,无法制作。显示一个持续时间为 3000 毫秒的提示消息 `language.makeFail`。
- 当 `data.code` 为 1 时,不需要预热。调用 `toPush` 函数,传入 `'pages/home/make.qml'`、`'make'` 和一个包含相关信息的对象。
// 鼠标滚轮事件 popup.onwheel = function (e) { e.preventDefault(); // 阻止默认滚轮行为处理页面滚动 scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例 scale = Math.max(scale, 0.1); // 最小缩放比例为0.1 img.style.transform = "scale(" + scale + ")"; }; // 键盘按键事件 document.onkeydown = function (e) { // 检查是否显示悬浮窗 if (isPopupVisible) { switch (e.keyCode) { case 38: // 上箭头键 // 同行之前一张图片 // 实现逻辑代码 break; case 40: // 下箭头键 // 同行之后一张图片 // 实现逻辑代码 break; } } }; overlay.onclick = function () { document.body.removeChild(popup); document.body.removeChild(overlay); isPopupVisible = false; // 设置悬浮窗显示状态为false }; imgContainer.appendChild(img); popup.appendChild(imgContainer); document.body.appendChild(popup); }添加进去后不能进行图片的切换
非常抱歉,我之前的回答有误导。为了实现在悬浮窗放大图片后使用键盘方向键进行同行之前上下张的切换,您需要在`showPopup`函数中添加一些代码。请使用以下代码替换您之前提供的代码:
```javascript
function showPopup(src) {
isPopupVisible = true; // 设置悬浮窗显示状态为true
var overlay = document.createElement("div");
overlay.style.position = "fixed";
overlay.style.top = "0";
overlay.style.left = "0";
overlay.style.width = "100%";
overlay.style.height = "100%";
overlay.style.backgroundColor = "rgba(0, 0, 0, 0.8)";
overlay.style.zIndex = "9998"; // 设置遮罩层的z-index低于悬浮窗,但高于其他元素
document.body.appendChild(overlay);
var popup = document.createElement("div");
popup.style.position = "fixed";
popup.style.top = "50%";
popup.style.left = "50%";
popup.style.transform = "translate(-50%, -50%)";
popup.style.backgroundColor = "rgba(0, 0, 0, 0.8)";
popup.style.zIndex = "9999";
popup.style.width = "75%"; // 设置悬浮窗宽度为页面宽度的75%
popup.style.height = "75%"; // 设置悬浮窗高度为页面高度的75%
popup.style.overflow = "hidden";
var imgContainer = document.createElement("div");
imgContainer.style.display = "flex";
imgContainer.style.justifyContent = "center";
imgContainer.style.alignItems = "center";
imgContainer.style.backgroundColor = "transparent";
imgContainer.style.width = "100%";
imgContainer.style.height = "100%";
var img = document.createElement("img");
img.src = src;
img.alt = "放大照片";
img.style.maxWidth = "100%";
img.style.maxHeight = "100%";
var images = []; // 存储所有图片的数组
var currentIndex = 0; // 当前显示的图片索引
// 添加图片到数组中
// 假设您有一个存储所有图片URL的数组,将其赋值给images变量
images.push("image1.jpg");
images.push("image2.jpg");
images.push("image3.jpg");
// ...
// 键盘按键事件
document.onkeydown = function (e) {
// 检查是否显示悬浮窗
if (isPopupVisible) {
switch (e.keyCode) {
case 38: // 上箭头键
currentIndex = (currentIndex - 1 + images.length) % images.length;
img.src = images[currentIndex];
break;
case 40: // 下箭头键
currentIndex = (currentIndex + 1) % images.length;
img.src = images[currentIndex];
break;
}
}
};
// 点击图片放大/缩小
img.onclick = function () {
scale += 0.1;
img.style.transform = "scale(" + scale + ")";
};
// 鼠标滚轮事件
popup.onwheel = function (e) {
e.preventDefault(); // 阻止默认滚轮行为处理页面滚动
scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例
scale = Math.max(scale, 0.1); // 最小缩放比例为0.1
img.style.transform = "scale(" + scale + ")";
};
overlay.onclick = function () {
document.body.removeChild(popup);
document.body.removeChild(overlay);
isPopupVisible = false; // 设置悬浮窗显示状态为false
};
imgContainer.appendChild(img);
popup.appendChild(imgContainer);
document.body.appendChild(popup);
}
```
请注意,上述代码假设您有一个存储所有图片URL的数组,并且使用上箭头键(keyCode为38)可切换到同行之前的一张图片,使用下箭头键(keyCode为40)可切换到同行之后的一张图片。您需要根据实际情况修改`images`数组中的URL和键盘事件的逻辑。
希望这次能帮到您!如果还有其他问题,请随时提问。
阅读全文
相关推荐
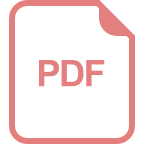
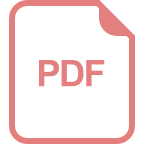
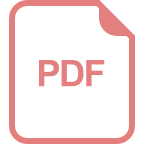
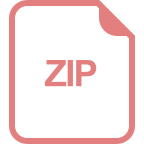
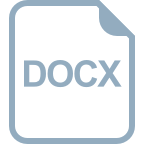
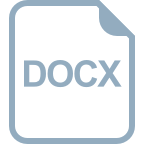
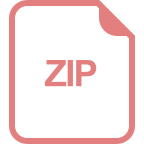
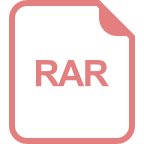
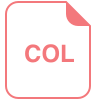
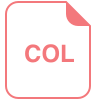
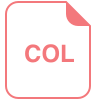
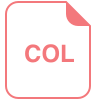
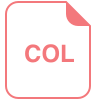
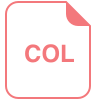
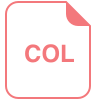
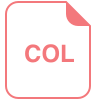
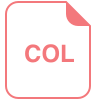