区域感知结构相似度损失函数tensorflow代码实现
时间: 2023-07-28 17:34:42 浏览: 113
区域感知结构相似度损失函数(Perceptual Structural Similarity Loss with Region-wise Attention)是一种改进的目标感知结构相似度损失函数,用于图像生成、风格迁移等任务中。其核心思想是在目标感知结构相似度损失的基础上,引入区域感知的注意力机制,以提高损失函数的鲁棒性和效果。下面是区域感知结构相似度损失函数的tensorflow代码实现:
```
import tensorflow as tf
from tensorflow.keras.applications.vgg16 import VGG16
# 加载预训练的VGG16模型
vgg16 = VGG16(include_top=False, weights='imagenet', input_shape=(None, None, 3))
vgg16.trainable = False
# 选择VGG16的某些层作为特征提取器
layers = ['block1_conv2', 'block2_conv2', 'block3_conv3', 'block4_conv3']
outputs = [vgg16.get_layer(name).output for name in layers]
model = tf.keras.Model(inputs=vgg16.input, outputs=outputs)
def region_aware_perceptual_structural_similarity_loss(y_true, y_pred):
# 提取目标图像和生成图像的特征表示
true_features = model(y_true)
pred_features = model(y_pred)
# 计算每个特征图的MSE损失,并乘以对应的注意力权重
loss = 0
for true_feature, pred_feature in zip(true_features, pred_features):
mse_loss = tf.keras.losses.MSE(true_feature, pred_feature)
attention = region_aware_attention(true_feature, pred_feature)
loss += mse_loss * attention
loss /= len(true_features)
return loss
def region_aware_attention(x, y, kernel_size=3):
# 计算特征图的均值和标准差
x_mean, x_var = tf.nn.moments(x, axes=[1, 2], keepdims=True)
y_mean, y_var = tf.nn.moments(y, axes=[1, 2], keepdims=True)
# 计算相似度矩阵
xy = tf.nn.conv2d(x * y, tf.ones((kernel_size, kernel_size, x.shape[-1], 1)), strides=[1, 1, 1, 1], padding='SAME')
xx = tf.nn.conv2d(x ** 2, tf.ones((kernel_size, kernel_size, x.shape[-1], 1)), strides=[1, 1, 1, 1], padding='SAME')
yy = tf.nn.conv2d(y ** 2, tf.ones((kernel_size, kernel_size, x.shape[-1], 1)), strides=[1, 1, 1, 1], padding='SAME')
sigma = tf.sqrt(xx * yy)
similarity = xy / sigma
# 计算区域感知的注意力权重
max_similarity = tf.reduce_max(similarity, axis=[1, 2], keepdims=True)
attention = tf.exp(similarity - max_similarity)
attention *= tf.cast(attention > 1e-5, tf.float32)
attention /= tf.reduce_sum(attention, axis=[1, 2], keepdims=True)
return attention
```
在上面的代码中,我们首先提取目标图像和生成图像的特征表示,然后对于每个特征图,计算其MSE损失,并乘以对应的区域感知的注意力权重。注意力权重的计算方法是先计算相似度矩阵,然后对相似度矩阵进行归一化处理,最后得到每个像素点的注意力权重。
阅读全文
相关推荐
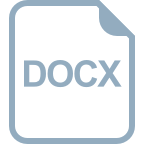

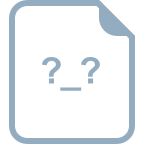
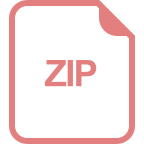
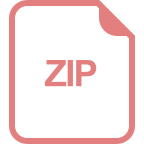
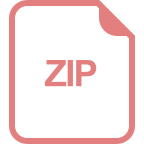
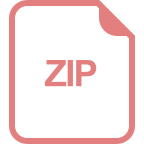
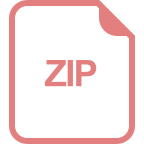
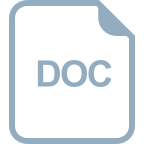
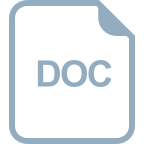
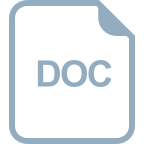
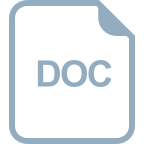
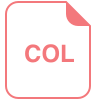
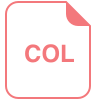
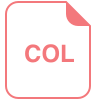
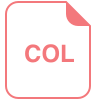

