实现MAX517驱动程序
时间: 2023-09-16 21:07:54 浏览: 57
MAX517是一款数字式可调电压/电流输出DAC芯片,其驱动程序的实现方式取决于所使用的控制器类型和编程语言。以下是一些常见的实现方式:
1. 使用Arduino控制器和Arduino IDE编程语言实现MAX517驱动程序:
```c++
#include <Wire.h>
#define MAX517_ADDRESS 0x2F
void setup() {
Wire.begin();
Serial.begin(9600);
}
void setVoltage(uint16_t voltage) {
uint8_t highByte = (voltage >> 8) & 0xFF;
uint8_t lowByte = voltage & 0xFF;
Wire.beginTransmission(MAX517_ADDRESS);
Wire.write(highByte);
Wire.write(lowByte);
Wire.endTransmission();
}
void loop() {
setVoltage(2048); // Set the output voltage to 50% of the reference voltage (VREF)
delay(1000);
setVoltage(4095); // Set the output voltage to the maximum value
delay(1000);
}
```
2. 使用Raspberry Pi控制器和Python编程语言实现MAX517驱动程序:
```python
import smbus
MAX517_ADDRESS = 0x2F
def set_voltage(voltage):
high_byte = (voltage >> 8) & 0xFF
low_byte = voltage & 0xFF
bus.write_i2c_block_data(MAX517_ADDRESS, high_byte, [low_byte])
if __name__ == '__main__':
bus = smbus.SMBus(1)
set_voltage(2048) # Set the output voltage to 50% of the reference voltage (VREF)
time.sleep(1)
set_voltage(4095) # Set the output voltage to the maximum value
time.sleep(1)
```
3. 使用STM32控制器和C语言实现MAX517驱动程序:
```c
#include "stm32f10x.h"
#include "i2c.h"
#define MAX517_ADDRESS 0x2F
void setVoltage(uint16_t voltage) {
uint8_t highByte = (voltage >> 8) & 0xFF;
uint8_t lowByte = voltage & 0xFF;
I2C_StartTransmission(MAX517_ADDRESS, I2C_Direction_Transmitter);
I2C_SendData(highByte);
I2C_SendData(lowByte);
I2C_StopTransmission();
}
int main(void) {
I2C_Configuration();
setVoltage(2048); // Set the output voltage to 50% of the reference voltage (VREF)
Delay(1000);
setVoltage(4095); // Set the output voltage to the maximum value
Delay(1000);
}
```
相关推荐
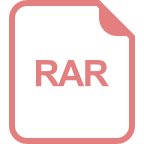











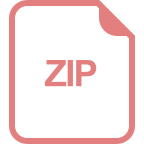