vue中被继承的组件使用setup,如何继承
时间: 2024-05-07 21:16:56 浏览: 336
一个被使用setup声明的组件呢?
你可以使用 `defineComponent` 函数来定义一个被使用 `setup` 声明的组件,并且在新组件的 `setup` 函数中使用 `extends` 来继承原组件的 `setup` 函数。
示例代码如下:
```
import { defineComponent } from 'vue';
import TheBaseComponent from './TheBaseComponent.vue';
export default defineComponent({
extends: TheBaseComponent,
setup(props, { attrs }) {
// 新组件的逻辑代码
},
});
```
其中,`TheBaseComponent` 是原组件的文件路径或者组件对象。通过 `extends` 继承原组件的 `setup` 函数,可以在新组件中重复利用原组件的逻辑。
注意,这种方式只适用于被使用 `setup` 声明的组件,如果原组件没有使用 `setup`,则需要按照正常的组件继承方式来继承组件。
相关问题
vue中被继承的组件使用了<script setup>,如何继承
在Vue中,可以使用`<script setup>`语法来简化组件的书写,在使用继承时,需要注意以下几点:
1. 父组件需要使用`<script>`标签来定义组件,而不能使用`<script setup>`
2. 子组件使用`<script setup>`语法定义组件,并使用`extends`关键字来继承父组件
3. 在子组件中,需要使用`defineProps`来定义与父组件传递的属性相对应的props,并使用`setup`函数来初始化组件的数据和方法
下面是一个简单的示例:
```vue
<!-- 父组件 -->
<template>
<div>
<h1>{{ title }}</h1>
<p>{{ content }}</p>
</div>
</template>
<script>
export default {
props: {
title: String,
content: String
}
}
</script>
<!-- 子组件 -->
<template>
<div>
<h2>{{ subtitle }}</h2>
<p>{{ subcontent }}</p>
</div>
</template>
<script setup extends="ParentComponent">
import { defineProps } from 'vue'
const props = defineProps({
subtitle: String,
subcontent: String
})
return {
...props
}
</script>
```
在子组件中,使用`extends`关键字来继承父组件,并使用`defineProps`定义子组件的props。在`setup`函数中,使用`...props`展开props,以便在子组件中可以直接访问父组件传递的属性。
vue3 中 继承ui组件
在 Vue3 中,可以通过使用 `defineComponent` 函数来定义一个组件,并使用 `extends` 关键字来继承另一个组件。例如,下面是一个使用 Element Plus 组件库中的 `ElButton` 组件作为父组件,并定义了一个名为 `MyButton` 的子组件的示例:
```javascript
import { defineComponent } from 'vue';
import { ElButton } from 'element-plus';
export default defineComponent({
name: 'MyButton',
extends: ElButton,
props: {
// 子组件的属性
},
setup(props, { attrs }) {
// 子组件的逻辑
},
// 子组件的模板
template: `
<el-button v-bind="$attrs" v-on="$listeners">
<slot></slot>
</el-button>
`
});
```
在上述代码中,`defineComponent` 函数返回一个组件选项对象,其中 `extends` 属性指定了父组件,`props` 属性定义了子组件的属性,`setup` 函数定义了子组件的逻辑,`template` 属性定义了子组件的模板。在模板中,使用了 `slot` 元素来插入子组件的内容。
通过这种方式,可以方便地继承和扩展现有的 UI 组件,从而快速构建复杂的界面。
阅读全文
相关推荐
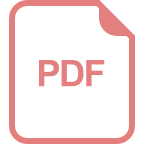
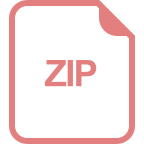
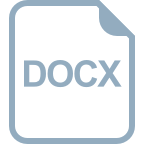
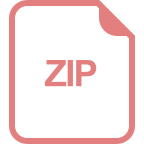
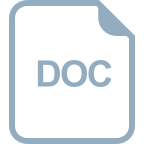
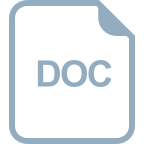
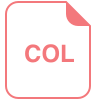










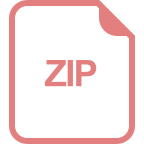