java代码实现一个单点登录更能
时间: 2023-11-11 14:48:52 浏览: 150
单点登录(Single Sign-On,简称SSO)是一种身份验证的机制,它允许用户使用一组凭据(例如用户名和密码)登录一次,然后在多个应用程序中进行访问而无需再次登录。在Java中实现单点登录可以采用以下步骤:
1. 配置一个共享的认证中心(Authentication Center),该认证中心用于验证用户的身份。可以使用OpenID Connect或OAuth 2.0等标准协议实现认证中心。
2. 在每个需要进行单点登录的应用程序中,集成认证中心的客户端库,该客户端库用于向认证中心发送认证请求并接收认证结果。
3. 当用户访问一个需要进行认证的应用程序时,该应用程序将重定向到认证中心,并向认证中心发送一个认证请求。
4. 认证中心验证用户的身份,并返回一个令牌(Token)给应用程序。
5. 应用程序使用该令牌来验证用户的身份,并在用户访问其他需要进行认证的应用程序时重复上述步骤。
以下是一个简单的Java代码示例,演示了如何使用Spring Security实现基于OAuth 2.0的单点登录:
1. 配置认证中心(在这里使用Google作为认证中心)
```java
@Configuration
@EnableOAuth2Client
public class OAuth2ClientConfig {
@Value("${google.clientId}")
private String clientId;
@Value("${google.clientSecret}")
private String clientSecret;
@Bean
public OAuth2ProtectedResourceDetails googleOAuth2Details() {
AuthorizationCodeResourceDetails details = new AuthorizationCodeResourceDetails();
details.setClientId(clientId);
details.setClientSecret(clientSecret);
details.setAccessTokenUri("https://accounts.google.com/o/oauth2/token");
details.setUserAuthorizationUri("https://accounts.google.com/o/oauth2/auth");
details.setScope(Arrays.asList("email", "profile"));
details.setPreEstablishedRedirectUri("http://localhost:8080/login/oauth2/code/google");
details.setUseCurrentUri(false);
return details;
}
@Bean
public OAuth2RestTemplate googleOAuth2RestTemplate(OAuth2ClientContext oauth2ClientContext) {
OAuth2RestTemplate restTemplate = new OAuth2RestTemplate(googleOAuth2Details(), oauth2ClientContext);
restTemplate.setMessageConverters(Arrays.asList(new MappingJackson2HttpMessageConverter()));
return restTemplate;
}
}
```
2. 配置应用程序的安全性
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private OAuth2ClientContext oauth2ClientContext;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/login**", "/webjars/**").permitAll()
.anyRequest().authenticated()
.and()
.logout()
.logoutSuccessUrl("/")
.permitAll()
.and()
.oauth2Login()
.loginPage("/login")
.authorizationEndpoint()
.baseUri("/login/oauth2/authorize")
.and()
.redirectionEndpoint()
.baseUri("/login/oauth2/code/*")
.and()
.userInfoEndpoint()
.userService(userService())
.and()
.tokenEndpoint()
.accessTokenResponseClient(accessTokenResponseClient())
.and()
.defaultSuccessURL("/home")
.failureUrl("/login?error");
}
@Bean
public OAuth2UserService<OAuth2UserRequest, OAuth2User> userService() {
return new DefaultOAuth2UserService();
}
@Bean
public OAuth2AccessTokenResponseClient<OAuth2AuthorizationCodeGrantRequest> accessTokenResponseClient() {
return new DefaultAuthorizationCodeTokenResponseClient();
}
}
```
3. 配置应用程序的授权服务器
```java
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Value("${spring.security.oauth2.client.registration.custom.client-id}")
private String clientId;
@Value("${spring.security.oauth2.client.registration.custom.client-secret}")
private String clientSecret;
@Autowired
private AuthenticationManager authenticationManager;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient(clientId)
.secret(clientSecret)
.authorizedGrantTypes("password", "refresh_token")
.scopes("read", "write")
.accessTokenValiditySeconds(3600)
.refreshTokenValiditySeconds(86400);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager);
}
}
```
使用以上代码示例,可以快速实现基于OAuth 2.0的单点登录功能。当然,实现SSO的方法有很多,开发者可以根据实际需求进行选择。
阅读全文
相关推荐
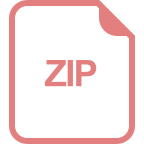
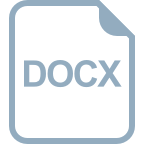
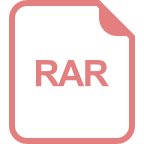
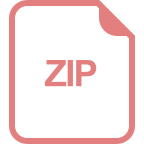
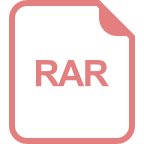
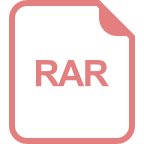
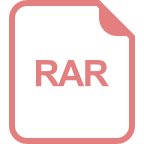
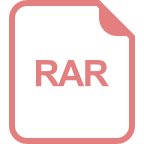
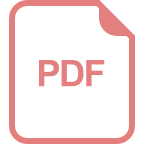
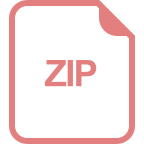
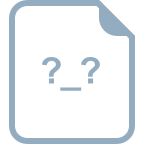
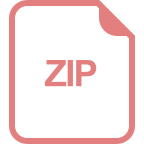
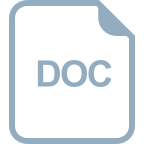
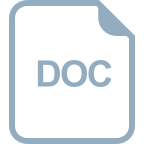