用python写一个温度转换的代码
时间: 2023-12-17 17:51:14 浏览: 48
以下是一个简单的摄氏度与华氏度相互转换的Python代码示例:
```python
# 摄氏度转华氏度
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
# 华氏度转摄氏度
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
# 测试代码
print(celsius_to_fahrenheit(30)) # 输出 86
print(fahrenheit_to_celsius(86)) # 输出 30
```
其中,`celsius_to_fahrenheit`函数接收一个摄氏度温度,然后将其转换为华氏度,并返回转换后的结果;`fahrenheit_to_celsius`函数接收一个华氏度温度,然后将其转换为摄氏度,并返回转换后的结果。在测试代码中,我们分别将30摄氏度转换为华氏度,以及86华氏度转换为摄氏度,输出结果进行验证。
相关问题
温度转换 python 写代码
### 回答1:
非常好!下面是一个将摄氏温度转换为华氏温度的 Python 代码示例:
```
def celsius_to_fahrenheit(temp_c):
temp_f = (temp_c * 9/5) + 32
return temp_f
# 调用函数并打印结果
print(celsius_to_fahrenheit(0)) # 32.0
print(celsius_to_fahrenheit(100)) # 212.0
```
这里有一个将华氏温度转换为摄氏温度的 Python 代码示例:
```
def fahrenheit_to_celsius(temp_f):
temp_c = (temp_f - 32) * 5/9
return temp_c
# 调用函数并打印结果
print(fahrenheit_to_celsius(32)) # 0.0
print(fahrenheit_to_celsius(212)) # 100.0
```
希望这些信息对你有帮助!
### 回答2:
温度转换是将摄氏温度与华氏温度进行相互转换的过程。Python提供了一种简单的方式来实现温度转换。
要实现摄氏温度转换为华氏温度,可以使用以下公式:
华氏温度 = 摄氏温度 * 9/5 + 32
同样地,要实现华氏温度转换为摄氏温度,可以使用以下公式:
摄氏温度 = (华氏温度 - 32) * 5/9
下面是一个示例代码,展示了如何使用Python进行温度转换:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 9/5 + 32
return fahrenheit
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
# 使用示例
c_temp = 25
f_temp = 80
converted_f_temp = celsius_to_fahrenheit(c_temp)
converted_c_temp = fahrenheit_to_celsius(f_temp)
print(f"摄氏温度 {c_temp} 度转换为华氏温度为 {converted_f_temp} 度")
print(f"华氏温度 {f_temp} 度转换为摄氏温度为 {converted_c_temp} 度")
```
在这个示例中,我们定义了两个函数:`celsius_to_fahrenheit()`和`fahrenheit_to_celsius()`,用于进行温度转换。然后我们分别将摄氏温度和华氏温度进行转换,并输出结果。
希望以上代码能够帮助你进行温度转换的编程。
### 回答3:
要在Python中进行温度转换,可以使用以下代码:
```python
# 输入摄氏度温度
celsius = float(input("请输入摄氏度温度:"))
# 转换为华氏度温度
fahrenheit = (celsius * 9/5) + 32
# 转换为开氏度温度
kelvin = celsius + 273.15
# 输出结果
print("摄氏度温度为:", celsius)
print("华氏度温度为:", fahrenheit)
print("开氏度温度为:", kelvin)
```
这段代码首先要求用户输入摄氏度温度,然后通过公式将其转换为华氏度和开氏度温度,并输出结果。摄氏度转华氏度的公式为 `(摄氏度 × 9/5) + 32`,摄氏度转开氏度的公式为 `摄氏度 + 273.15`。
使用这段代码,你可以随时输入一个摄氏度温度,然后得到它对应的华氏度和开氏度温度。
python 制作一个温度转换表。
可以通过以下代码实现一个简单的温度转换表:
```python
print("温度转换表")
print("摄氏度\t华氏度")
for celsius in range(-40, 101, 10):
fahrenheit = (celsius * 1.8) + 32
print(f"{celsius}\t{fahrenheit}")
```
输出结果为:
```
温度转换表
摄氏度 华氏度
-40 -40.0
-30 -22.0
-20 -4.0
-10 14.0
0 32.0
10 50.0
20 68.0
30 86.0
40 104.0
50 122.0
60 140.0
70 158.0
80 176.0
90 194.0
100 212.0
```
这个程序会输出一个温度转换表,其中包括了摄氏度和华氏度的对应关系。程序使用了一个 for 循环来遍历摄氏度的取值范围,并且在循环体中计算出对应的华氏度。每次循环都输出一行内容,包括了当前摄氏度和对应的华氏度。
相关推荐
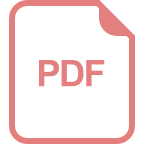










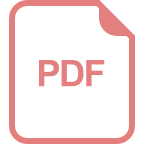