基于boost库,使用c++语言编写一个阻塞模式接收udp组播的代码
时间: 2024-03-07 10:50:07 浏览: 94
下面是一个基于Boost库,使用C++语言编写的阻塞模式接收UDP组播数据的代码```c++
#include <iostream>
#include <boost/asio.hpp>
using boost::asio::ip::udp;
int main()
{
try
{
boost::asio::io_context io_context;
// 创建接收端点
udp::endpoint listen_endpoint(boost::asio::ip::address::from_string("239.255.255.250"), 1900);
// 创建socket并绑定到接收端点
udp::socket socket(io_context);
socket.open(listen_endpoint.protocol());
socket.set_option(boost::asio::ip::udp::socket::reuse_address(true));
socket.bind(listen_endpoint);
std::cout << "Listening on " << listen_endpoint << std::endl;
for (;;)
{
char data[1024];
udp::endpoint sender_endpoint;
size_t length = socket.receive_from(boost::asio::buffer(data, 1024), sender_endpoint);
std::cout << "Received " << length << " bytes from " << sender_endpoint << std::endl;
std::cout << "Data: " << data << std::endl;
}
}
catch (std::exception& e)
{
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
```
这个程序会监听239.255.255.250:1900的UDP组播数据包。当有数据包到达时,程序会输出信息并打印接收到的数据。
需要注意的是,这个程序是阻塞模式的,也就是说,当没有数据包到达时,程序会一直阻塞在socket.receive_from()函数处。如果需要实现非阻塞模式,可以使用Boost的异步操作机制。
阅读全文
相关推荐

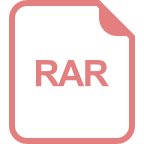
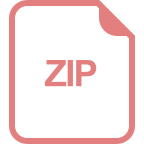
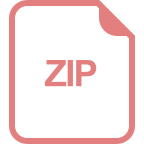
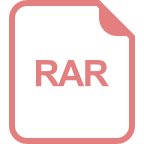
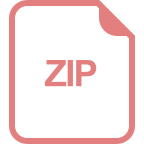
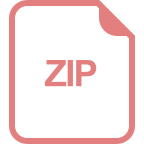
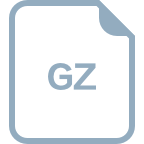
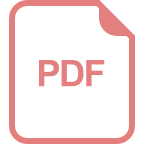
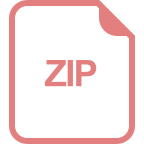
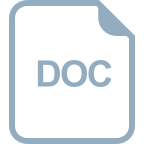
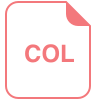
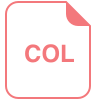


