androidstudio使用Viewpage和tablayout实现滑动效果
时间: 2024-02-02 17:05:16 浏览: 167
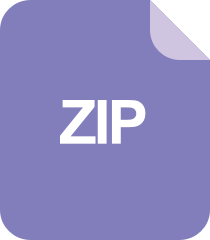
Android Studio 使用ViewPager + Fragment实现滑动菜单Tab效果
好的,下面是使用ViewPager和TabLayout实现滑动效果的步骤:
1.在布局文件中添加ViewPager和TabLayout组件
```xml
<android.support.design.widget.TabLayout
android:id="@+id/tabLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorPrimary"
app:tabMode="scrollable"
app:tabTextColor="@android:color/white"
app:tabSelectedTextColor="@android:color/white"
app:tabIndicatorColor="@android:color/white"
app:tabIndicatorHeight="3dp" />
<android.support.v4.view.ViewPager
android:id="@+id/viewPager"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2.在Java代码中初始化ViewPager和TabLayout组件
```java
ViewPager viewPager = findViewById(R.id.viewPager);
TabLayout tabLayout = findViewById(R.id.tabLayout);
MyFragmentPagerAdapter adapter = new MyFragmentPagerAdapter(getSupportFragmentManager());
viewPager.setAdapter(adapter);
tabLayout.setupWithViewPager(viewPager);
```
3.实现FragmentPagerAdapter类,用于管理ViewPager中的Fragment
```java
public class MyFragmentPagerAdapter extends FragmentPagerAdapter {
private String[] titles = {"Tab 1", "Tab 2", "Tab 3"};
public MyFragmentPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
switch (position) {
case 0:
return new Fragment1();
case 1:
return new Fragment2();
case 2:
return new Fragment3();
default:
return null;
}
}
@Override
public int getCount() {
return titles.length;
}
@Nullable
@Override
public CharSequence getPageTitle(int position) {
return titles[position];
}
}
```
4.实现三个Fragment类,用于显示在ViewPager中的内容
```java
public class Fragment1 extends Fragment {
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment1_layout, container, false);
return view;
}
}
public class Fragment2 extends Fragment {
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment2_layout, container, false);
return view;
}
}
public class Fragment3 extends Fragment {
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment3_layout, container, false);
return view;
}
}
```
5.创建三个布局文件fragment1_layout.xml、fragment2_layout.xml、fragment3_layout.xml,用于显示在ViewPager中的内容
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:text="Fragment 1"
android:textSize="30sp"
android:gravity="center"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
```
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:text="Fragment 2"
android:textSize="30sp"
android:gravity="center"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
```
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:text="Fragment 3"
android:textSize="30sp"
android:gravity="center"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
```
以上就是使用ViewPager和TabLayout实现滑动效果的步骤,希望对你有帮助。
阅读全文
相关推荐
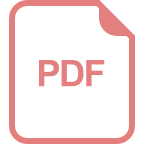
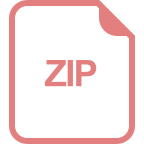

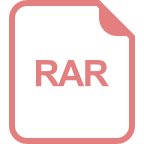
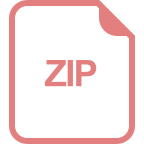
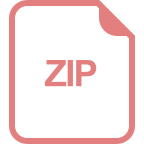
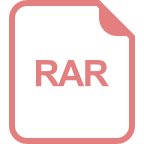
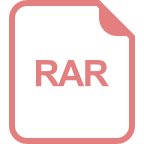
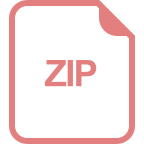
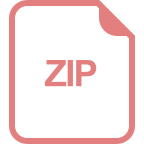
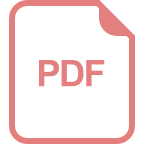
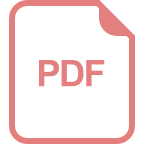
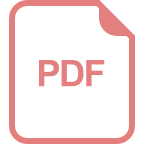
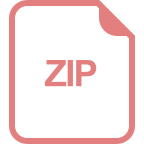
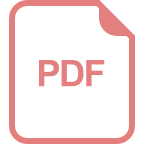
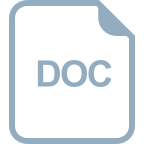