能用python初始化一些数据,让我运行上面的搜索算法吗?
时间: 2023-12-22 12:05:13 浏览: 24
当然可以!你可以使用 Python 编写一个脚本来初始化数据,然后运行搜索算法。以下是一个简单的示例,可以帮助你快速入门。
```python
# 导入搜索算法需要的模块
from queue import PriorityQueue
import math
# 初始化数据
start = (0, 0) # 起点坐标
end = (4, 4) # 终点坐标
obstacles = [(2, 2), (3, 1), (3, 3)] # 障碍物坐标
# 定义计算两点距离的函数
def distance(point1, point2):
x1, y1 = point1
x2, y2 = point2
return math.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
# 定义启发式函数
def heuristic(point):
return distance(point, end)
# 定义搜索算法
def a_star(start, end, obstacles):
frontier = PriorityQueue()
frontier.put(start, 0)
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while not frontier.empty():
current = frontier.get()
if current == end:
break
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
next_point = (current[0] + dx, current[1] + dy)
if next_point in obstacles:
continue
new_cost = cost_so_far[current] + distance(current, next_point)
if next_point not in cost_so_far or new_cost < cost_so_far[next_point]:
cost_so_far[next_point] = new_cost
priority = new_cost + heuristic(next_point)
frontier.put(next_point, priority)
came_from[next_point] = current
return came_from, cost_so_far
# 运行搜索算法
came_from, cost_so_far = a_star(start, end, obstacles)
# 打印结果
print("came_from:", came_from)
print("cost_so_far:", cost_so_far)
```
在这个示例中,我们使用了 A* 搜索算法来寻找从起点到终点的最短路径。我们首先初始化了起点、终点和障碍物的坐标,然后定义了一个计算两点距离的函数和一个启发式函数。接着,我们使用了优先队列来实现 A* 算法,并在算法运行结束后打印了结果。
当然,你可以根据自己的需要修改示例代码,来满足你的具体需求。
相关推荐
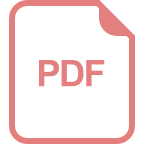
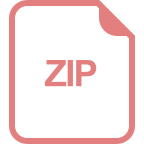
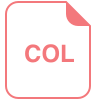
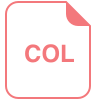

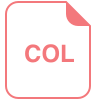
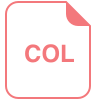









