用c++实现一组点的x或y坐标相同,对应的y或x坐标的和等于一个固定值
时间: 2024-03-23 21:39:16 浏览: 21
以下是用C++实现一组点的x或y坐标相同,对应的y或x坐标的和等于一个固定值的例子:
```c++
#include <iostream>
#include <unordered_map>
#include <vector>
using namespace std;
vector<pair<int, int>> findPairs(int arr[][2], int n, int sum, bool isXCoord) {
vector<pair<int, int>> result;
unordered_map<int, int> hash;
for (int i = 0; i < n; i++) {
int coord = isXCoord ? arr[i][0] : arr[i][1];
int otherCoord = sum - coord;
if (hash.find(otherCoord) != hash.end()) { // Found matching coordinate
int count = hash[otherCoord];
for (int j = 0; j < count; j++) { // Add all matching coordinates
result.push_back(isXCoord ? make_pair(i, hash[otherCoord + j]) : make_pair(hash[otherCoord + j], i));
}
}
hash[coord]++;
}
return result;
}
int main() {
int arr[][2] = {{1, 2}, {3, 4}, {5, 6}, {7, 8}, {9, 10}};
int n = sizeof(arr) / sizeof(arr[0]);
int sum = 10;
vector<pair<int, int>> xMatches = findPairs(arr, n, sum, true);
vector<pair<int, int>> yMatches = findPairs(arr, n, sum, false);
cout << "X Matches:\n";
for (auto& p : xMatches) {
cout << "(" << arr[p.first][0] << ", " << arr[p.first][1] << ") and (" << arr[p.second][0] << ", " << arr[p.second][1] << ")\n";
}
cout << "\nY Matches:\n";
for (auto& p : yMatches) {
cout << "(" << arr[p.first][0] << ", " << arr[p.first][1] << ") and (" << arr[p.second][0] << ", " << arr[p.second][1] << ")\n";
}
return 0;
}
```
这个程序首先实现了一个用于查找坐标对的函数`findPairs`,它接受一个二维数组,数组大小,一个固定的值,以及一个指示要匹配x坐标或y坐标的布尔值。该函数使用一个哈希表来存储已经访问的坐标,然后遍历数组中的每个坐标,检查是否存在匹配的坐标。如果找到了匹配的坐标,它会将所有匹配的坐标对添加到一个结果向量中。
主函数中,我们首先定义了一个包含坐标的二维数组和一个固定的值。然后,我们调用`findPairs`函数两次,一次用于匹配x坐标,一次用于匹配y坐标。最后,我们循环遍历每个匹配的坐标对,并将它们打印到控制台上。
相关推荐
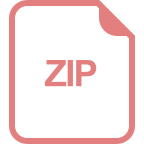
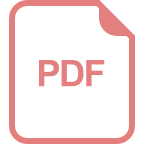














