针对 boss 直聘网站,使用 Scrapy 框架爬取全国热门城市大数据、数据分析、数据挖掘、机器学习、人工智能等相关岗位的招聘信息,将数据清洗后存入mysql数据库中。提供详解和代码
时间: 2024-06-09 20:10:46 浏览: 22
首先,我们需要搭建Scrapy环境。可以使用Anaconda进行安装,在Anaconda Prompt中使用以下命令:
```
conda install scrapy
```
接下来,我们需要创建一个新的Scrapy项目,可以在命令行中使用以下命令:
```
scrapy startproject boss
```
这将创建一个名为“boss”的新项目。
接下来,我们需要定义我们要爬取的网站。在项目文件夹中,创建一个名为“spiders”的文件夹,并在其中创建一个名为“boss_spider.py”的Python文件。在该文件中,我们需要编写爬虫代码。以下是一个简单的爬虫,爬取boss直聘网站的数据:
```python
import scrapy
class BossSpider(scrapy.Spider):
name = 'boss'
allowed_domains = ['www.zhipin.com']
start_urls = ['https://www.zhipin.com/c101010100/?query=%E6%95%B0%E6%8D%AE%E5%88%86%E6%9E%90&page=1']
def parse(self, response):
for job in response.css('.job-list ul li'):
yield {
'title': job.css('.job-title::text').get(),
'salary': job.css('.red::text').get(),
'location': job.css('.job-area::text').get(),
'experience': job.css('.job-pub-time::text').get(),
'education': job.css('.job-pub-time::text').get(),
'company': job.css('.company-text .name::text').get(),
'industry': job.css('.company-text p::text').get(),
}
next_page = response.css('.page a.next::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
```
在上述代码中,我们首先定义了一个名为“BossSpider”的类,该类继承了Scrapy的Spider类。在该类中,我们定义了要爬取的网站域名和起始URL。在parse()函数中,我们使用CSS选择器来选择所需数据并将其存储到字典中。然后,我们使用response.follow()方法来跟随下一页链接并继续爬取数据。
接下来,我们需要将爬取的数据存储到MySQL数据库中。我们可以使用Python中的mysql-connector库来实现这一点。以下是一个简单的代码示例:
```python
import mysql.connector
class BossPipeline:
def __init__(self):
self.conn = mysql.connector.connect(
user='root',
password='password',
host='localhost',
database='boss'
)
self.cursor = self.conn.cursor()
def process_item(self, item, spider):
sql = 'INSERT INTO jobs (title, salary, location, experience, education, company, industry) VALUES (%s, %s, %s, %s, %s, %s, %s)'
values = (
item['title'],
item['salary'],
item['location'],
item['experience'],
item['education'],
item['company'],
item['industry']
)
self.cursor.execute(sql, values)
self.conn.commit()
return item
def close_spider(self, spider):
self.conn.close()
```
在上述代码中,我们定义了一个名为“BossPipeline”的类,该类是一个Scrapy管道。在该类中,我们首先建立了一个MySQL连接,并在process_item()函数中执行了INSERT语句以将数据存储到数据库中。最后,在close_spider()函数中关闭了MySQL连接。
要启用管道,请打开项目中的“settings.py”文件,并添加以下代码:
```python
ITEM_PIPELINES = {
'boss.pipelines.BossPipeline': 300,
}
```
在上述代码中,我们将管道的优先级设置为300,以确保它在其他管道之前运行。
现在,我们可以在命令行中运行以下命令来启动爬虫并将数据存储到MySQL数据库中:
```
scrapy crawl boss
```
以上是一个简单的爬取boss直聘网站并将数据存储到MySQL数据库的流程和代码示例。但是需要注意的是,爬虫程序需要遵守网站的 robots.txt 规则,同时需要设置合理的请求头信息,以避免被封禁。
相关推荐
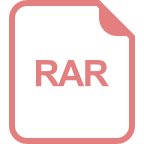
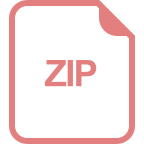














