使用trimesh库表示一个面
时间: 2024-05-11 15:13:52 浏览: 196
要使用trimesh库表示一个面,需要先创建一个三角形网格对象,然后将面添加到该对象中。以下是一个示例代码,其中包含一个三角形网格对象和一个面的添加:
```python
import trimesh
# 创建三角形网格对象
mesh = trimesh.Trimesh()
# 添加一个面
face_vertices = [[0,0,0], [1,0,0], [0,1,0]] # 三个顶点的坐标
face_indices = [[0,1,2]] # 三个顶点在mesh.vertices中的索引
mesh.add_geometry(face_vertices, face_indices)
```
在上面的代码中,我们首先创建了一个空的三角形网格对象,然后定义了一个三角形面的三个顶点坐标和在网格对象中的索引。最后,我们使用`add_geometry`方法将面添加到网格对象中。
请注意,这只是一个简单的示例,实际应用中可能需要更多的面和顶点来表示复杂的几何形状。
相关问题
trimesh不透光
trimesh是一个Python库,用于处理三维几何数据,特别是三角形网格。如果你想要创建一个不透光(也称为“不透明”或“填充模式”)的模型,trimesh提供了一个属性`face_colors`或`vertices_colors`来指定每个面或顶点的颜色。通过设置颜色为完全不透明(例如,白色或黑色),你可以让物体看起来不透光。
例如,在trimesh中,你可以这样做:
```python
import trimesh
# 创建一个trimesh对象
mesh = trimesh.primitives.Box()
# 设置所有面为不透明
mesh.face_colors = [0, 0, 0, 255] # 四通道颜色,其中alpha通道值为255表示完全不透明
# 显示不透光的模型
scene = trimesh.Scene([mesh])
scene.show()
```
基于VCGLIB库的三角网格精简算法及示例代码
基于VCGLIB库的三角网格精简算法的示例代码如下:
```
#include <iostream>
#include <vector>
#include <vcg/complex/complex.h>
#include <vcg/complex/algorithms/clean.h>
#include <vcg/complex/algorithms/update/topology.h>
#include <vcg/complex/algorithms/update/flag.h>
#include <vcg/complex/algorithms/update/selection.h>
#include <vcg/complex/algorithms/local_optimization.h>
#include <vcg/space/intersection3.h>
using namespace vcg;
using namespace std;
class MyVertex;
class MyEdge;
class MyFace;
struct MyUsedTypes : public UsedTypes<
Use<MyVertex>::AsVertexType,
Use<MyEdge>::AsEdgeType,
Use<MyFace>::AsFaceType>{};
class MyVertex : public Vertex<MyUsedTypes, vertex::Coord3f, vertex::Normal3f, vertex::VFAdj> {
public:
float importance; // 顶点重要性
};
class MyEdge : public Edge<MyUsedTypes> {};
class MyFace : public Face<MyUsedTypes, face::VertexRef, face::Normal3f, face::VFAdj, face::FFAdj> {};
class MyMesh : public vcg::tri::TriMesh<vector<MyVertex>, vector<MyFace>> {};
// 计算顶点的重要性
void ComputeVertexImportance(MyMesh &mesh) {
for (auto &v : mesh.vert) {
v.importance = 0.0f;
for (auto &vf : v.vf) {
auto &f = *vf;
float a = (f.P(1) - f.P(0)).Norm();
float b = (f.P(2) - f.P(1)).Norm();
float c = (f.P(0) - f.P(2)).Norm();
float s = (a + b + c) * 0.5f;
float area = sqrt(s * (s - a) * (s - b) * (s - c));
v.importance += area;
}
}
}
// 计算三角面片质量
float ComputeFaceQuality(const MyFace &f) {
auto &v0 = *f.V(0);
auto &v1 = *f.V(1);
auto &v2 = *f.V(2);
auto q0 = (v1.P() - v0.P()).Norm();
auto q1 = (v2.P() - v1.P()).Norm();
auto q2 = (v0.P() - v2.P()).Norm();
auto s = (q0 + q1 + q2) / 2.0f;
auto area = sqrt(s * (s - q0) * (s - q1) * (s - q2));
auto h0 = 2.0f * area / q0;
auto h1 = 2.0f * area / q1;
auto h2 = 2.0f * area / q2;
auto quality = min(min(h0, h1), h2);
return quality;
}
// 删除冗余三角面片
void SimplifyMesh(MyMesh &mesh, float qualityThreshold, float importanceThreshold) {
// 更新拓扑信息
tri::UpdateTopology<MyMesh>::FaceFace(mesh);
tri::UpdateTopology<MyMesh>::VertexFace(mesh);
// 标记所有三角面片为未选中状态
tri::UpdateFlags<MyMesh>::FaceClear(mesh);
tri::UpdateFlags<MyMesh>::VertexClear(mesh);
// 计算三角面片质量和顶点重要性
for (auto &f : mesh.face)
f.Q() = ComputeFaceQuality(f);
ComputeVertexImportance(mesh);
// 根据质量和重要性进行三角面片选择
tri::UpdateSelection<MyMesh>::FaceFromQuality(mesh, qualityThreshold);
tri::UpdateSelection<MyMesh>::VertexFromFaceLoose<MyVertex>(mesh);
for (auto &v : mesh.vert)
if (v.IsV() && v.importance < importanceThreshold)
v.SetS();
// 删除未选中的三角面片和顶点
tri::Clean<MyMesh>::RemoveFaces(mesh);
tri::Clean<MyMesh>::RemoveIsolatedVertices(mesh);
}
int main(int argc, char *argv[]) {
if (argc != 4) {
cout << "Usage: " << argv[0] << " input.obj output.obj qualityThreshold importanceThreshold" << endl;
return 1;
}
float qualityThreshold = atof(argv[3]);
float importanceThreshold = atof(argv[4]);
MyMesh mesh;
// 读取模型数据
if (vcg::tri::io::Importer<MyMesh>::Open(mesh, argv[1]) != 0) {
// 精简模型
SimplifyMesh(mesh, qualityThreshold, importanceThreshold);
// 输出模型数据
if (vcg::tri::io::Exporter<MyMesh>::Save(mesh, argv[2]) != 0) {
cout << "Save mesh failed." << endl;
return 1;
}
} else {
cout << "Read mesh failed." << endl;
return 1;
}
return 0;
}
```
该示例代码实现了基于VCGLIB库的三角网格精简算法,其主要步骤为:
1.定义MyVertex、MyEdge和MyFace类,分别用于表示顶点、边和面。这些类继承自VCGLIB库中的顶点、边和面类,并添加了一些自定义属性。
2.定义MyMesh类,用于表示三角网格。该类继承自VCGLIB库中的三角网格类,并指定其顶点、面和边类型为MyVertex、MyFace和MyEdge。
3.实现ComputeVertexImportance函数,用于计算每个顶点的重要性。
4.实现ComputeFaceQuality函数,用于计算每个三角面片的质量。
5.实现SimplifyMesh函数,用于精简三角网格。该函数的主要步骤为:
(1)更新三角网格的拓扑信息。
(2)计算每个三角面片的质量和每个顶点的重要性。
(3)根据质量和重要性进行三角面片和顶点的选择。
(4)删除未选中的三角面片和顶点。
6.在main函数中,读取输入模型数据,调用SimplifyMesh函数进行精简,并将精简后的模型数据输出到文件中。
需要注意的是,该示例代码中的qualityThreshold和importanceThreshold参数分别表示三角面片的质量阈值和顶点的重要性阈值。如果三角面片的质量或顶点的重要性低于对应的阈值,则会被删除。
阅读全文
相关推荐
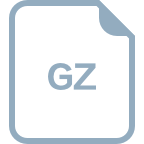
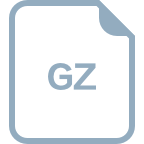
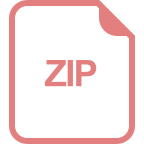
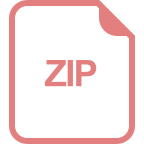
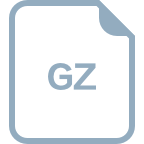
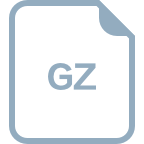
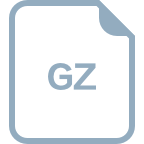
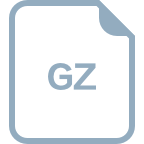
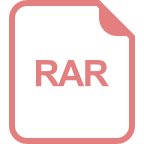
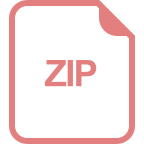
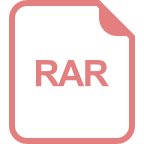
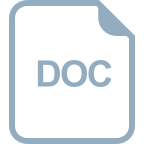
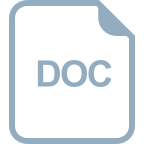
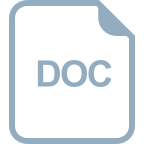
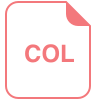
