c++ stl的hashmap怎么实现
时间: 2023-09-03 18:11:10 浏览: 169
C++ STL中的HashMap是通过哈希表实现的,下面是它的基本实现方法:
1. 定义一个桶数组,数组元素是链表。每个元素都是一个存储键值对的链表,相同哈希值的键值对存储在同一个链表中。
2. 在HashMap中提供一个哈希函数,用于将键映射到桶数组中的某个位置。
3. 插入元素时,先通过哈希函数计算出元素应该存储的桶的位置,然后将元素插入到对应的链表中。
4. 查找元素时,先通过哈希函数计算出元素存储的桶的位置,然后遍历对应的链表查找元素。
5. 删除元素时,先通过哈希函数计算出元素存储的桶的位置,然后在对应的链表中查找并删除元素。
下面是HashMap的基本实现代码:
```c++
#include <iostream>
#include <unordered_map>
using namespace std;
int main()
{
unordered_map<int, string> myMap;
// 插入元素
myMap.insert(make_pair(1, "hello"));
myMap.insert(make_pair(2, "world"));
// 查找元素
cout << myMap[1] << endl; // 输出 hello
// 删除元素
myMap.erase(2);
return 0;
}
```
上面的代码使用了C++ STL中的unordered_map,它是一个基于哈希表实现的关联容器。通过它,我们可以方便地实现哈希表的功能。
相关问题
c++实现hashmap
C++中可以使用STL中的unordered_map来实现hashmap,也可以手动实现hashmap。
以下是一个简单的手动实现hashmap的示例代码:
```c++
#include <iostream>
#include <vector>
#include <list>
using namespace std;
class HashMap {
private:
vector<list<pair<int, int>>> table; // 存储桶
int capacity; // hashmap容量
int size; // hashmap当前大小
// 获取哈希码
int hash(int key) {
return key % capacity;
}
public:
HashMap(int capacity) {
this->capacity = capacity;
this->size = 0;
table.resize(capacity);
}
// 插入键值对
void put(int key, int value) {
int index = hash(key);
auto &bucket = table[index]; // 获取对应桶
for (auto &node : bucket) { // 查找是否已存在key
if (node.first == key) {
node.second = value; // 更新value
return;
}
}
bucket.emplace_back(key, value); // 插入新节点
size++; // 更新大小
}
// 获取key对应的value
int get(int key) {
int index = hash(key);
auto &bucket = table[index]; // 获取对应桶
for (auto &node : bucket) { // 查找是否存在key
if (node.first == key) {
return node.second; // 返回对应value
}
}
return -1; // 未找到对应key
}
// 删除key对应的节点
void remove(int key) {
int index = hash(key);
auto &bucket = table[index]; // 获取对应桶
for (auto iter = bucket.begin(); iter != bucket.end(); iter++) { // 查找是否存在key
if (iter->first == key) {
bucket.erase(iter); // 移除节点
size--; // 更新大小
return;
}
}
}
// 获取hashmap当前大小
int getSize() {
return size;
}
};
int main() {
HashMap hashMap(10);
hashMap.put(1, 10);
hashMap.put(2, 20);
hashMap.put(11, 110);
hashMap.put(21, 210);
cout << hashMap.get(1) << endl; // 10
cout << hashMap.get(11) << endl; // 110
cout << hashMap.get(3) << endl; // -1
hashMap.remove(2);
cout << hashMap.getSize() << endl; // 3
return 0;
}
```
在该示例代码中,使用vector来存储桶,每个桶是一个链表,存储键值对。在插入、获取、删除操作时,先根据key的哈希值计算出对应的桶,再在桶中查找是否存在对应的键值对。如果存在,则进行更新或移除操作;如果不存在,则进行插入操作。
c++实现HashMap
HashMap(哈希表)是一种常用的数据结构,可以用来实现快速的查找、插入和删除操作。在C++中,可以使用STL中的unordered_map实现HashMap,也可以自己手动实现。
以下是一个C++手动实现的HashMap代码示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
class HashMap {
private:
vector<pair<int, int>>* data;
int size;
int hash(int key) {
return key % size;
}
public:
HashMap(int s) {
size = s;
data = new vector<pair<int, int>>[size];
}
void put(int key, int value) {
int index = hash(key);
for (auto& pair : data[index]) {
if (pair.first == key) {
pair.second = value;
return;
}
}
data[index].push_back(make_pair(key, value));
}
int get(int key) {
int index = hash(key);
for (auto& pair : data[index]) {
if (pair.first == key) {
return pair.second;
}
}
return -1;
}
void remove(int key) {
int index = hash(key);
auto& pairs = data[index];
for (auto it = pairs.begin(); it != pairs.end(); it++) {
if (it->first == key) {
pairs.erase(it);
return;
}
}
}
~HashMap() {
delete[] data;
}
};
int main() {
HashMap map(10);
map.put(1, 1);
map.put(2, 2);
cout << map.get(1) << endl; // 输出1
cout << map.get(3) << endl; // 输出-1
map.put(2, 3);
cout << map.get(2) << endl; // 输出3
map.remove(2);
cout << map.get(2) << endl; // 输出-1
return 0;
}
```
在上面的代码中,我们使用了一个vector数组来存储数据。每个vector元素是一个pair,第一个元素是key,第二个元素是value。在put操作时,我们使用hash函数来计算key对应的index,然后遍历该index对应的vector,如果找到了相同的key,则更新value,否则加入一个新的pair。在get操作时,同样使用hash函数计算key对应的index,然后遍历该index对应的vector,查找相同的key,并返回其对应的value。在remove操作时,也是先计算key对应的index,然后查找相同的key,并将其从vector中删除。
需要注意的是,在删除操作时,我们使用了auto&来引用pairs变量,这样可以避免在遍历过程中对pairs进行拷贝,提高了效率。
阅读全文
相关推荐







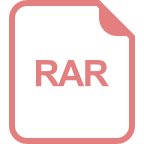






