public class IdiomProcessor { public static void main(String[] args) { // 定义输入文件路径和输出文件路径 String inputFile ="D:\yy\java\成语接龙\成语大全\去重后.txt"; String outputFile = "D:\yy\java\成语接龙\成语大全\全量成语处理后.txt"; try (BufferedReader reader = new BufferedReader(new FileReader(inputFile)); BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) { // 定义成语列表和成语末尾字与开头字相同的数量Map List<String> idiomList = new ArrayList<>(); Map<Character, Integer> countMap = new HashMap<>(); // 读取每一行成语信息 String line; while ((line = reader.readLine())!= null) { if (line.trim().isEmpty()) { continue; // 跳过空行 } if (line.contains("拼音:")) { // 处理包含指定内容的行 System.out.println("我动了"); // 将成语与其他信息分割 String[] parts = line.split("拼音:"); // 将成语加入成语列表 String idiom = parts[0]; idiomList.add(idiom); // 计算成语末尾字与开头字相同的数量 char lastChar = idiom.charAt(idiom.length() - 1); countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1); // 将原来的信息每一条加上count后输出到另一个文件 String newLine = line + "可接:" + countMap.get(lastChar) + "\n"; writer.write(newLine); } } } catch (IOException e) { e.printStackTrace(); } } }由于代码的最后一个字会重复,所以用Map记录时,输出的count有错,如何改正
时间: 2024-03-13 19:46:07 浏览: 27
可以在计算成语末尾字与开头字相同的数量时,排除掉最后一个字即可。
具体的做法是,将计算 count 的代码段:
```
char lastChar = idiom.charAt(idiom.length() - 1);
countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1);
```
修改成:
```
char lastChar = idiom.charAt(idiom.length() - 1);
char firstChar = parts[1].charAt(0); // 获取成语的拼音
if (lastChar != firstChar) { // 判断是否重复
countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1);
}
```
这样,就可以排除掉最后一个字,避免重复计数,同时保证了成语末尾字与开头字相同的数量的正确性。
相关问题
public class IdiomProcessor { public static void main(String[] args) { // 定义输入文件路径和输出文件路径 String inputFile ="D:\yy\java\成语接龙\成语大全\去重后.txt"; String outputFile = "D:\yy\java\成语接龙\成语大全\全量成语处理后.txt"; try (BufferedReader reader = new BufferedReader(new FileReader(inputFile)); BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) { // 定义成语列表和成语末尾字与开头字相同的数量Map List<String> idiomList = new ArrayList<>(); Map<Character, Integer> countMap = new HashMap<>(); // 读取每一行成语信息 String line; while ((line = reader.readLine())!= null) { if (line.trim().isEmpty()) { continue; // 跳过空行 } if (line.contains("拼音:")) { // 处理包含指定内容的行 System.out.println("我动了"); // 将成语与其他信息分割 String[] parts = line.split("拼音:"); // 将成语加入成语列表 String idiom = parts[0]; idiomList.add(idiom); // 计算成语末尾字与开头字相同的数量 char lastChar = idiom.charAt(idiom.length() - 1); countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1); // 将原来的信息每一条加上count后输出到另一个文件 String newLine = line + "可接:" + countMap.get(lastChar) + "\n"; writer.write(newLine); } } } catch (IOException e) { e.printStackTrace(); } } }由于代码在用Map统计lastChar时不同成语的末尾字会相同,所以用Map记录时,输出的count有错,如何改正
可以使用一个 `Map<String, Integer>` 来记录每个成语的末尾字与开头字相同的数量。
具体的做法是,在计算成语末尾字与开头字相同的数量时,将 `countMap` 改为 `idiomCountMap`,并且将 key 从 `Character` 改为 `String`,其 value 记录的是成语末尾字与开头字相同的数量。
修改后的代码如下:
```
public class IdiomProcessor {
public static void main(String[] args) {
// 定义输入文件路径和输出文件路径
String inputFile = "D:\\yy\\java\\成语接龙\\成语大全\\去重后.txt";
String outputFile = "D:\\yy\\java\\成语接龙\\成语大全\\全量成语处理后.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(inputFile));
BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) {
// 定义成语列表和成语末尾字与开头字相同的数量Map
List<String> idiomList = new ArrayList<>();
Map<String, Integer> idiomCountMap = new HashMap<>();
// 读取每一行成语信息
String line;
while ((line = reader.readLine()) != null) {
if (line.trim().isEmpty()) {
continue; // 跳过空行
}
if (line.contains("拼音:")) { // 处理包含指定内容的行
System.out.println("我动了");
// 将成语与其他信息分割
String[] parts = line.split("拼音:");
// 将成语加入成语列表
String idiom = parts[0];
idiomList.add(idiom);
// 计算成语末尾字与开头字相同的数量
char lastChar = idiom.charAt(idiom.length() - 1);
String key = lastChar + parts[1].substring(0, 1);
idiomCountMap.put(key, idiomCountMap.getOrDefault(key, 0) + 1);
// 将原来的信息每一条加上count后输出到另一个文件
String newLine = line + "可接:" + idiomCountMap.get(key) + "\n";
writer.write(newLine);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这样,就可以正确统计每个不同成语的末尾字与开头字相同的数量了。
import java.io.File;import javax.sound.sampled.AudioInputStream;import javax.sound.sampled.AudioSystem;import javax.sound.sampled.Clip;public class BackgroundMusic { public static void main(String[] args) { try { File file = new File("music.wav"); // 音乐文件路径 AudioInputStream audioStream = AudioSystem.getAudioInputStream(file); Clip clip = AudioSystem.getClip(); clip.open(audioStream); clip.loop(Clip.LOOP_CONTINUOUSLY); // 循环播放 } catch (Exception e) { e.printStackTrace(); } }}为什么播放不了
有可能是因为音乐文件路径不正确导致的。请确保 `music.wav` 文件在当前工程目录下,或者使用绝对路径指定文件路径。另外,也需要检查你的音频文件是否符合 Java 支持的格式。Java 只支持一些特定的音频格式,例如 WAV、AIFF、AU 等,如果你使用的音频格式不符合要求,也会导致无法播放。你可以尝试使用其他格式的音频文件测试一下。另外,如果你使用的是 Eclipse 等 IDE 进行开发,也需要检查你的 IDE 是否设置了正确的类路径和资源路径。
相关推荐
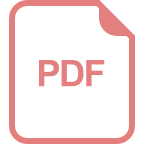
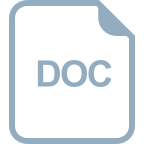
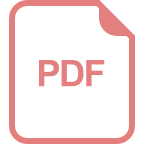














