Java中的sql更新语句
时间: 2024-05-16 22:16:52 浏览: 13
在Java中执行SQL更新语句的步骤如下:
1. 获取数据库连接:使用JDBC API中的DriverManager.getConnection()方法获取数据库连接。
2. 创建Statement对象:使用Connection对象的createStatement()方法创建一个Statement对象。
3. 执行SQL更新语句:使用Statement对象的executeUpdate()方法执行SQL更新语句。
4. 关闭Statement对象和数据库连接:使用Statement对象的close()方法关闭Statement对象,使用Connection对象的close()方法关闭数据库连接。
以下是一个Java中执行SQL更新语句的示例代码:
```
import java.sql.*;
public class UpdateExample {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 创建Statement对象
stmt = conn.createStatement();
// 执行SQL更新语句
String sql = "UPDATE users SET age = 20 WHERE name = 'Tom'";
int rowsUpdated = stmt.executeUpdate(sql);
System.out.println("Rows updated: " + rowsUpdated);
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
// 关闭Statement对象和数据库连接
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
注意:在实际应用中,为了防止SQL注入攻击,应该使用PreparedStatement对象代替Statement对象执行SQL更新语句。
相关推荐
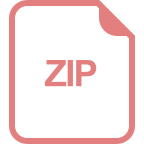
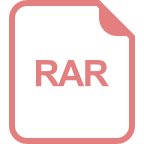
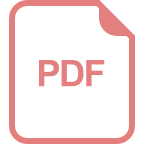














