通过共享内存的方式,cpu0和cpu1以指令方式建立通信的代码
时间: 2024-02-25 11:52:42 浏览: 23
下面是一个示例代码,展示了如何在CPU0和CPU1之间通过共享内存实现指令通信:
首先,在共享内存区域中定义一个变量:
```
#define SHARED_MEM_SIZE 1024
struct shared_mem {
// 定义共享内存中的变量
int flag;
char instruction[SHARED_MEM_SIZE];
};
```
然后,在CPU0中初始化共享内存区域,并向其中写入指令:
```
// 打开共享内存
int fd = shm_open("/my_shared_mem", O_CREAT | O_RDWR, 0666);
if (fd == -1) {
perror("shm_open");
exit(-1);
}
// 设置共享内存的大小
if (ftruncate(fd, sizeof(struct shared_mem)) == -1) {
perror("ftruncate");
exit(-1);
}
// 将共享内存映射到进程的地址空间
struct shared_mem *mem = mmap(NULL, sizeof(struct shared_mem), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (mem == MAP_FAILED) {
perror("mmap");
exit(-1);
}
// 在共享内存中写入指令
mem->flag = 1; // 设置标志位为1表示有指令可执行
strcpy(mem->instruction, "do_something()");
```
最后,在CPU1中执行共享内存中的指令:
```
// 打开共享内存
int fd = shm_open("/my_shared_mem", O_CREAT | O_RDWR, 0666);
if (fd == -1) {
perror("shm_open");
exit(-1);
}
// 将共享内存映射到进程的地址空间
struct shared_mem *mem = mmap(NULL, sizeof(struct shared_mem), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (mem == MAP_FAILED) {
perror("mmap");
exit(-1);
}
// 等待CPU0写入指令
while (mem->flag == 0) {
sleep(1); // 等待1秒钟
}
// 执行共享内存中的指令
char *instruction = mem->instruction;
system(instruction);
// 修改标志位并清空共享内存
mem->flag = 0; // 设置标志位为0表示已执行完指令
strcpy(mem->instruction, ""); // 清空共享内存中的指令
```
以上代码仅供参考,具体实现还需要根据您的需求进行调整。
相关推荐
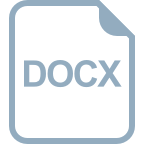
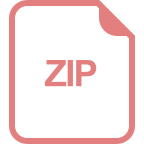
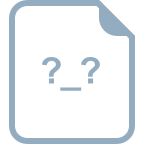














