python使用svm两种图像分类代码
时间: 2023-07-30 10:07:26 浏览: 205
以下是两个使用Python的Scikit-learn库实现SVM图像分类的示例代码:
### 代码1:使用HOG特征进行图像分类
HOG(Histogram of Oriented Gradients)是一种图像特征提取方法,常用于图像分类和物体检测。以下是一个使用HOG特征进行图像分类的示例代码:
```python
import cv2
import numpy as np
from sklearn import svm
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from skimage.feature import hog
from skimage import data, exposure
# 加载图像数据集
dataset = []
labels = []
for i in range(0, 10):
for j in range(0, 100):
img = cv2.imread("data/" + str(i) + "_" + str(j) + ".png", cv2.IMREAD_GRAYSCALE)
dataset.append(img)
labels.append(i)
# 提取HOG特征
hog_features = []
for img in dataset:
fd = hog(img, orientations=8, pixels_per_cell=(8, 8), cells_per_block=(1, 1), visualize=False)
hog_features.append(fd)
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(hog_features, labels, test_size=0.3, random_state=0)
# 创建SVM分类器
clf = svm.SVC(kernel='linear', C=1)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试数据
y_pred = clf.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:",accuracy)
# 显示测试图片和预测结果
for i in range(0, 10):
img = cv2.imread("data/" + str(i) + "_0.png", cv2.IMREAD_GRAYSCALE)
fd, hog_image = hog(img, orientations=8, pixels_per_cell=(8, 8), cells_per_block=(1, 1), visualize=True)
hog_image_rescaled = exposure.rescale_intensity(hog_image, in_range=(0, 10))
plt.subplot(2, 5, i+1)
plt.imshow(hog_image_rescaled, cmap=plt.cm.gray)
plt.title("Predict:" + str(clf.predict([fd])[0]))
plt.show()
```
这个示例代码使用了一个包含10个类别的图像数据集,并将其划分为训练集和测试集。然后,使用HOG特征提取方法对图像进行特征提取,并使用SVM分类器对训练集进行训练。最后,代码还展示了测试图片和预测结果。
### 代码2:使用颜色直方图进行图像分类
颜色直方图是一种常用的图像特征提取方法,用于描述图像中颜色的分布情况。以下是一个使用颜色直方图进行图像分类的示例代码:
```python
import cv2
import numpy as np
from sklearn import svm
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 加载图像数据集
dataset = []
labels = []
for i in range(0, 10):
for j in range(0, 100):
img = cv2.imread("data/" + str(i) + "_" + str(j) + ".png")
dataset.append(img)
labels.append(i)
# 提取颜色直方图特征
color_features = []
for img in dataset:
hist = cv2.calcHist([img], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256])
hist = cv2.normalize(hist, hist).flatten()
color_features.append(hist)
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(color_features, labels, test_size=0.3, random_state=0)
# 创建SVM分类器
clf = svm.SVC(kernel='linear', C=1)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试数据
y_pred = clf.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:",accuracy)
# 显示测试图片和预测结果
for i in range(0, 10):
img = cv2.imread("data/" + str(i) + "_0.png")
hist = cv2.calcHist([img], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256])
hist = cv2.normalize(hist, hist).flatten()
plt.subplot(2, 5, i+1)
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
plt.title("Predict:" + str(clf.predict([hist])[0]))
plt.show()
```
这个示例代码同样使用了一个包含10个类别的图像数据集,并将其划分为训练集和测试集。然后,使用颜色直方图特征提取方法对图像进行特征提取,并使用SVM分类器对训练集进行训练。最后,代码还展示了测试图片和预测结果。
相关推荐
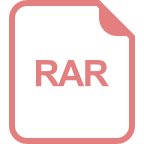
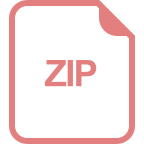














