ofdrw 签名 代码
时间: 2023-07-03 13:25:11 浏览: 44
OFDRW提供了Java语言的API来实现OFD文档的签名,以下是OFDRW签名的基本代码实现:
```java
// 创建OFD文档实例
OFDDocument ofd = new OFDDocument();
// 加载OFD文档
ofd.open("example.ofd");
// 创建数字签名实例
Signature signature = new Signature();
// 设置签名证书
signature.setCert("example.pfx", "123456");
// 设置签名位置和大小
signature.setSignaturePosition(100, 100, 200, 100);
// 对OFD文档进行签名
signature.sign(ofd);
// 保存签名后的OFD文档
ofd.save("example_signed.ofd");
```
其中,`example.ofd`是需要签名的OFD文档文件,`example.pfx`是签名证书文件,`123456`是证书密码。`setSignaturePosition()`方法用来设置签名的位置和大小,可以根据实际需要进行调整。`sign()`方法会对OFD文档进行签名,签名后的OFD文档会保存到`example_signed.ofd`文件中。
相关问题
ofdrw OFD 签名
OFD(Open Financial Dialog)是一种开放式的金融对话协议,可以让金融机构和第三方服务提供商之间进行安全、高效的数据交换。OFD中的签名机制是用来验证数据完整性和真实性的重要方法。OFDRW是OFD的一个子集,主要涉及OFD的文档相关功能,包括OFD文档的创建、编辑、签名等。OFDRW中的签名机制可以通过数字证书来实现,确保OFD文档的安全性和可靠性。
ofd电子签章 ofdrw代码实现
以下是使用OFDRW进行OFD电子签章的Java代码示例:
```java
import org.ofdrw.core.annotation.Annotations;
import org.ofdrw.core.annotation.pageannot.Annot;
import org.ofdrw.core.annotation.pageannot.Appearance;
import org.ofdrw.core.annotation.pageannot.CT_Annot;
import org.ofdrw.core.basicStructure.pageObj.layer.block.CT_Layer;
import org.ofdrw.core.basicStructure.pageObj.layer.block.PathObject;
import org.ofdrw.core.basicStructure.pageObj.layer.block.TextObject;
import org.ofdrw.core.basicStructure.pageObj.layer.type.ImageObject;
import org.ofdrw.core.basicStructure.pageObj.layer.type.PageBlockType;
import org.ofdrw.core.basicStructure.pageObj.layer.type.TextCode;
import org.ofdrw.core.basicStructure.res.CT_MultiMedia;
import org.ofdrw.core.basicStructure.res.Res;
import org.ofdrw.core.basicStructure.res.resources.MultiMedias;
import org.ofdrw.core.basicStructure.res.resources.Page;
import org.ofdrw.core.basicStructure.res.resources.Pages;
import org.ofdrw.core.basicStructure.res.resources.PublicRes;
import org.ofdrw.core.basicStructure.res.resources.Template;
import org.ofdrw.core.basicStructure.res.resources.Templates;
import org.ofdrw.core.basicType.ST_Box;
import org.ofdrw.core.pageDescription.CT_PageBlock;
import org.ofdrw.core.pageDescription.color.color.CT_Color;
import org.ofdrw.core.text.TextCodeExt;
import org.ofdrw.font.FontName;
import org.ofdrw.layout.OFDDoc;
import org.ofdrw.layout.PageLayout;
import org.ofdrw.layout.element.*;
import org.ofdrw.layout.element.canvas.DrawContext;
import org.ofdrw.layout.element.canvas.ImageFormObject;
import org.ofdrw.layout.element.canvas.Path;
import org.ofdrw.layout.element.canvas.TextCodePoint;
import org.ofdrw.layout.engine.ImageEngine;
import org.ofdrw.layout.engine.ResLocator;
import org.ofdrw.pkg.container.OFDEntry;
import org.ofdrw.reader.OFDReader;
import org.ofdrw.sign.SignUtils;
import org.ofdrw.sign.signContainer.SESV4Container;
import org.ofdrw.sign.signContainer.SignedData;
import org.ofdrw.sign.stamppos.NormalStampPos;
import org.ofdrw.sign.stamppos.StampPos;
import org.ofdrw.sign.verify.container.OFDSignedContainerVerify;
import org.ofdrw.sign.verify.container.SignedDataValidateResult;
import org.ofdrw.sign.verify.exceptions.ContainerNotFoundException;
import org.ofdrw.sign.verify.exceptions.SignatureValidateException;
import org.ofdrw.sign.verify.exceptions.SignatureVerifyException;
import org.ofdrw.sign.verify.exceptions.SealValidateException;
import org.ofdrw.sign.verify.signature.OFDSeal;
import org.ofdrw.sign.verify.signature.SignedValue;
import org.ofdrw.sign.verify.signature.SignedValueType;
import org.ofdrw.sign.verify.signature.exceptions.InvalidSignedValueException;
import org.ofdrw.sign.verify.signature.exceptions.SealIDNotFoundException;
import org.ofdrw.sign.verify.signature.exceptions.SealNotFoundException;
import org.ofdrw.sign.verify.signature.exceptions.SignatureNotFoundException;
import org.ofdrw.sign.verify.signature.exceptions.VersionNotSupportException;
import org.ofdrw.sign.verify.time.AuthorityInfo;
import org.ofdrw.sign.verify.time.TimeProtocolVerifier;
import org.ofdrw.simpleType.PageAreaType;
import org.ofdrw.simpleType.SizeType;
import org.ofdrw.simpleType.ST_Array;
import org.ofdrw.simpleType.ST_ID;
import org.ofdrw.simpleType.ST_Loc;
import org.ofdrw.simpleType.ST_RefID;
import org.ofdrw.simpleType.ST_Version;
import org.ofdrw.simpleType.Severity;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.security.GeneralSecurityException;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
public class OFDSignatureDemo {
/**
* 电子签章
*
* @param srcPath 原OFD文件路径
* @param dstPath 签章后OFD文件路径
* @param sealImgPath 签章图片路径
* @param certPath 数字证书路径
* @param password 数字证书密码
* @throws IOException
* @throws GeneralSecurityException
*/
public static void sign(String srcPath, String dstPath, String sealImgPath, String certPath, String password) throws IOException, GeneralSecurityException {
// 创建OFDDoc对象
OFDDoc ofdDoc = new OFDDoc(new PageLayout(PageAreaType.A4, SizeType.A4));
// 加载OFD文件
OFDReader reader = new OFDReader(srcPath);
reader.getConfig().setResCache(new ResLocator() {
@Override
public Res getRes(Path path) throws IOException {
try (OFDEntry entry = reader.get(path)) {
return entry.getOfd().getDocumentRes().getPublicRes().getRes(path);
}
}
});
reader.getConfig().setImageCache(new ImageEngine() {
@Override
public BufferedImage getImage(Path path) throws IOException {
try (OFDEntry entry = reader.get(path)) {
return ImageIO.read(entry.getStream());
}
}
});
ofdDoc.getPageTree().addAll(reader.getPageList());
// 创建签章对象
Image img = ImageIO.read(new File(sealImgPath));
ST_Box boundary = new ST_Box(0, 0, img.getWidth(null), img.getHeight(null));
ImageObject imageObject = new ImageObject(boundary, new ST_Loc("Res_0"));
CT_Color color = new CT_Color(0, 0, 0);
TextCode textCode = new TextCode(TextCodeExt.of("签章文字"));
TextObject textObject = new TextObject(new ST_Box(0, 0, 100, 20), color, textCode);
ST_Box position = new ST_Box(100, 100, 200, 200);
CT_Annot ctAnnot = new CT_Annot();
ctAnnot.setType(Annotations.ANNOT_TYPE_STAMP); // 签章类型
ctAnnot.setName("签章名称");
ctAnnot.setRect(position);
ctAnnot.setFringe(false);
ctAnnot.setCreator("OFDRW");
ctAnnot.setLastModDate(OffsetDateTime.now());
ctAnnot.setAppearance(new Appearance(new Annot(new ST_RefID("Res_1"))));
ctAnnot.setPageRef(ofdDoc.getPageTree().get(0).getOfdPage().getBaseLoc());
// 设置签章位置
StampPos stampPos = new NormalStampPos(position.getMinX(), position.getMinY(), position.getMaxX(), position.getMaxY(), 0);
// 对OFD文件进行签章
SESV4Container sesv4Container = new SESV4Container();
sesv4Container.setSignMethod(SignUtils.SignMethod.Nonvisible);
sesv4Container.setDigestMethod(SignUtils.DigestMethod.SHA256);
sesv4Container.setCertPath(certPath);
sesv4Container.setPassword(password);
sesv4Container.setSignedValueGenerator((digestAlgorithm, signedData) -> {
SignedValue signedValue = new SignedValue();
signedValue.setSignedValueType(SignedValueType.Seal);
signedValue.setIndex(1);
signedValue.setSignedData(signedData);
signedValue.setCertDigest(new byte[1][1]);
signedValue.setDigestAlgorithm(digestAlgorithm);
signedValue.setSignatureAlgorithm("1.2.840.113549.1.1.11"); // SHA256withRSA
signedValue.setSeal((new OFDSeal(imageObject, textObject, ctAnnot, stampPos)).toofdByteBuffer());
return signedValue;
});
ofdDoc.addSign(sesv4Container);
// 保存签章后的OFD文件
ofdDoc.save(dstPath);
ofdDoc.close();
}
/**
* 验证电子签章
*
* @param filePath 需要验证的OFD文件路径
* @param trustedCertsDir 可信数字证书路径
* @throws IOException
* @throws ContainerNotFoundException
* @throws SignatureNotFoundException
* @throws SignatureValidateException
* @throws SealNotFoundException
* @throws SealIDNotFoundException
* @throws InvalidSignedValueException
* @throws VersionNotSupportException
* @throws CertificateException
* @throws SealValidateException
* @throws SignatureVerifyException
*/
public static void verify(String filePath, String trustedCertsDir) throws IOException, ContainerNotFoundException, SignatureNotFoundException, SignatureValidateException, SealNotFoundException, SealIDNotFoundException, InvalidSignedValueException, VersionNotSupportException, CertificateException, SealValidateException, SignatureVerifyException {
// 加载OFD文件
OFDReader reader = new OFDReader(filePath);
// 验证数字签名
OFDSignedContainerVerify verifier = new OFDSignedContainerVerify(reader);
verifier.setTrustedCertDir(trustedCertsDir);
SignedDataValidateResult result = verifier.verify();
if (result.isSignatureValid() && result.isSealValid()) {
System.out.println("电子签章验证通过");
} else {
System.out.println("电子签章验证失败");
}
}
public static void main(String[] args) throws IOException, GeneralSecurityException {
String srcPath = "src/main/resources/test.ofd";
String dstPath = "src/main/resources/test-signed.ofd";
String sealImgPath = "src/main/resources/seal.png";
String certPath = "src/main/resources/test.pfx";
String password = "123456";
// 电子签章
sign(srcPath, dstPath, sealImgPath, certPath, password);
// 验证电子签章
verify(dstPath, "src/main/resources/certs");
}
}
```
注意,该示例中使用的数字证书为测试证书,仅供演示使用。在实际应用中应使用合法有效的数字证书,并且签章过程需要遵循相关法律法规和标准规范。
相关推荐
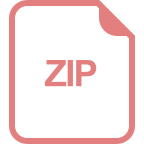
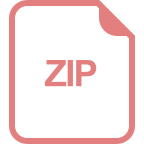












