C++将一个文件复制到另一个文件夹内
时间: 2023-09-01 09:07:45 浏览: 1712
你可以使用C++中的标准文件流(fstream)来实现将一个文件复制到另一个文件夹内的操作。具体步骤如下:
1. 打开源文件和目标文件
使用 `std::ifstream` 打开源文件,使用 `std::ofstream` 打开目标文件。如下所示:
```cpp
std::ifstream sourceFile("source.txt", std::ios::binary);
std::ofstream destFile("destination\\dest.txt", std::ios::binary);
```
其中,`source.txt` 是源文件的路径和名称,`destination\dest.txt` 是目标文件的路径和名称,要注意目标文件夹必须已经存在。
2. 读取源文件并写入目标文件
使用 `read()` 方法从源文件读取数据,使用 `write()` 方法将数据写入目标文件。如下所示:
```cpp
char buffer[1024];
while (sourceFile.read(buffer, sizeof(buffer))) {
destFile.write(buffer, sourceFile.gcount());
}
destFile.write(buffer, sourceFile.gcount());
```
其中,`buffer` 是用于存储数据的缓冲区,`sizeof(buffer)` 表示每次读取的最大字节数,`sourceFile.gcount()` 表示上一次读取的实际字节数。
3. 关闭文件流
完成文件复制后,需要关闭文件流以释放资源。如下所示:
```cpp
sourceFile.close();
destFile.close();
```
完整的代码如下:
```cpp
#include <fstream>
int main() {
std::ifstream sourceFile("source.txt", std::ios::binary);
std::ofstream destFile("destination\\dest.txt", std::ios::binary);
char buffer[1024];
while (sourceFile.read(buffer, sizeof(buffer))) {
destFile.write(buffer, sourceFile.gcount());
}
destFile.write(buffer, sourceFile.gcount());
sourceFile.close();
destFile.close();
return 0;
}
```
这样就可以将一个文件复制到另一个文件夹内了。
阅读全文
相关推荐









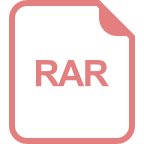




