写出用遗传算法解决考虑碳排放和运输成本的柔性作业车间调度问题的代码
时间: 2024-05-16 19:15:54 浏览: 17
这是一个比较复杂的问题,需要考虑的因素比较多。以下是一个简单的遗传算法的代码示例,可供参考:
```
import random
# 定义基因编码长度
GENE_LENGTH = 20
# 定义种群大小
POPULATION_SIZE = 50
# 定义迭代次数
MAX_ITERATIONS = 100
# 定义交叉概率和变异概率
CROSSOVER_RATE = 0.7
MUTATION_RATE = 0.1
# 定义车间数和任务数
NUM_WORKSHOPS = 5
NUM_JOBS = 10
# 定义车间间运输成本矩阵和任务加工时间矩阵
TRANSPORT_COST = [[0, 10, 20, 30, 40],
[10, 0, 15, 25, 35],
[20, 15, 0, 18, 28],
[30, 25, 18, 0, 12],
[40, 35, 28, 12, 0]]
PROCESS_TIME = [[10, 20, 30, 40, 50],
[20, 30, 40, 50, 60],
[30, 40, 50, 60, 70],
[40, 50, 60, 70, 80],
[50, 60, 70, 80, 90],
[60, 70, 80, 90, 100],
[70, 80, 90, 100, 110],
[80, 90, 100, 110, 120],
[90, 100, 110, 120, 130],
[100, 110, 120, 130, 140]]
# 定义染色体类
class Chromosome:
def __init__(self, gene):
self.gene = gene
self.fitness = 0
# 计算适应度函数
def calculate_fitness(self):
fitness = 0
for i in range(NUM_JOBS):
workshop = self.gene[i]
time = PROCESS_TIME[i][workshop]
fitness += time
if i > 0:
prev_workshop = self.gene[i-1]
transport_cost = TRANSPORT_COST[prev_workshop][workshop]
fitness += transport_cost
self.fitness = fitness
# 执行基因交叉
def crossover(self, other):
if random.random() < CROSSOVER_RATE:
crossover_point = random.randint(0, GENE_LENGTH - 1)
new_gene = self.gene[:crossover_point] + other.gene[crossover_point:]
return Chromosome(new_gene)
else:
return None
# 执行基因变异
def mutate(self):
if random.random() < MUTATION_RATE:
mutation_point = random.randint(0, GENE_LENGTH - 1)
new_gene = self.gene.copy()
new_gene[mutation_point] = random.randint(0, NUM_WORKSHOPS - 1)
return Chromosome(new_gene)
else:
return None
# 初始化种群
def initialize_population():
population = []
for i in range(POPULATION_SIZE):
gene = [random.randint(0, NUM_WORKSHOPS - 1) for j in range(NUM_JOBS)]
chromosome = Chromosome(gene)
chromosome.calculate_fitness()
population.append(chromosome)
return population
# 选择优秀的染色体
def select_chromosomes(population):
sorted_population = sorted(population, key=lambda x: x.fitness)
return sorted_population[:POPULATION_SIZE // 2]
# 定义遗传算法主函数
def genetic_algorithm():
# 初始化种群
population = initialize_population()
# 迭代
for i in range(MAX_ITERATIONS):
# 选择优秀的染色体
elites = select_chromosomes(population)
# 执行基因交叉和变异
children = []
while len(children) < POPULATION_SIZE // 2:
parent1 = random.choice(elites)
parent2 = random.choice(elites)
child = parent1.crossover(parent2)
if child:
child.mutate()
child.calculate_fitness()
children.append(child)
# 合并新一代种群
population = elites + children
# 输出最优解
best_chromosome = min(population, key=lambda x: x.fitness)
print(f"Iteration {i+1}: best fitness = {best_chromosome.fitness}, gene = {best_chromosome.gene}")
# 执行遗传算法
genetic_algorithm()
```
上述代码中,我们使用一个基因编码长度为 20 的染色体来表示调度方案,其中每个基因表示一个任务应该被分配到哪个车间。我们使用交叉和变异操作来产生新一代种群,并使用适应度函数计算每个染色体的适应度。在迭代过程中,我们输出每一代中最优解的适应度和基因编码。
相关推荐
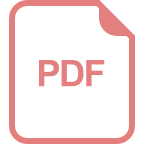














