请使用Java编程异步多线程和同步多线程的例子
时间: 2023-12-16 22:04:59 浏览: 80
好的,我可以为您提供Java编程异步多线程和同步多线程的例子。
首先,让我们来看看Java编程中异步多线程的例子:
```java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class AsyncThreadExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> {
// 异步线程执行的操作
System.out.println("异步线程执行了该操作");
});
executor.shutdown();
}
}
```
在上面的代码中,我们使用Java的Executor框架创建了一个单线程的线程池对象executor。然后我们使用submit()方法提交了一个异步执行的任务,该任务使用lambda表达式的形式定义。最后我们关闭了线程池。
现在,让我们来看看Java编程中同步多线程的例子:
```java
public class SyncThreadExample {
public static void main(String[] args) {
Thread thread1 = new Thread(new MyRunnable());
Thread thread2 = new Thread(new MyRunnable());
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("所有线程都已完成");
}
private static class MyRunnable implements Runnable {
@Override
public void run() {
synchronized (this) {
// 同步执行的代码块
System.out.println("线程" + Thread.currentThread().getName() + "正在执行该操作");
try {
Thread.sleep(500); // 并让线程睡眠500毫秒
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
```
在上面的代码中,我们创建了两个线程thread1和thread2,并在主线程中启动它们。在MyRunnable类的run()方法中,我们使用synchronized关键字锁定了当前线程,使线程同步执行其中的代码块。
接着,我们使用join()方法等待所有线程完成执行,最后输出所有线程都已完成。
希望这个例子对您有所帮助!
阅读全文
相关推荐
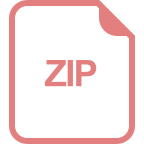
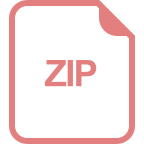
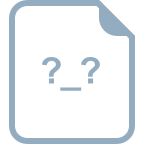
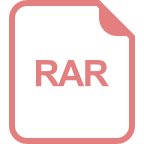
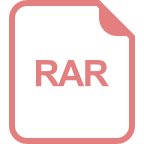
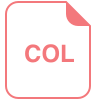
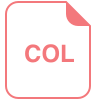
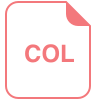
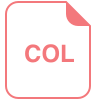
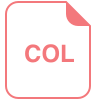
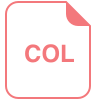
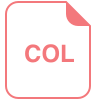
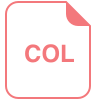
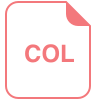
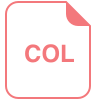
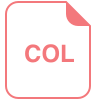