可以帮我用C#语言在visual studio 中编写一个CAD二次开发.net代码,运行该代码生产dll文件可以使用户在CAD中加载该dll文件后选择两条直线,由用户输入直线等分数量,最后连接对应等分点生成相应等分线
时间: 2024-04-01 17:34:35 浏览: 147
好的,我可以帮你编写这个CAD二次开发的.NET代码。首先,你需要在Visual Studio中安装AutoCAD的开发环境,然后创建一个新的Class Library项目。接下来,请按照以下步骤操作:
1. 添加对AutoCAD的引用
在项目中,右键点击“引用”,选择“添加引用”,在弹出的对话框中选择“浏览”,找到AutoCAD安装目录下的acmgd.dll、acdbmgd.dll、accoremgd.dll和acdbmgdbrep.dll等文件,添加它们作为项目的引用。
2. 添加命名空间
在代码文件中添加以下命名空间:
```csharp
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
using Autodesk.AutoCAD.EditorInput;
```
3. 编写命令函数
在代码文件中添加一个命令函数,用于处理用户在CAD中输入的命令。这个函数需要使用[CommandMethod]特性进行标记,以便AutoCAD能够识别它作为命令。
```csharp
[CommandMethod("MyCommand")]
public static void MyCommand()
{
// TODO: Add your code here
}
```
4. 获取用户选择的直线
在命令函数中,使用Editor类的GetEntity方法获取用户选择的两条直线。
```csharp
Editor ed = Application.DocumentManager.MdiActiveDocument.Editor;
PromptEntityOptions peo = new PromptEntityOptions("\nSelect first line: ");
peo.SetRejectMessage("\nInvalid object type.");
peo.AddAllowedClass(typeof(Line), true);
PromptEntityResult per1 = ed.GetEntity(peo);
if (per1.Status != PromptStatus.OK) return;
ObjectId id1 = per1.ObjectId;
PromptEntityOptions peo2 = new PromptEntityOptions("\nSelect second line: ");
peo2.SetRejectMessage("\nInvalid object type.");
peo2.AddAllowedClass(typeof(Line), true);
peo2.AddAllowedClass(typeof(Curve), true);
PromptEntityResult per2 = ed.GetEntity(peo2);
if (per2.Status != PromptStatus.OK) return;
ObjectId id2 = per2.ObjectId;
```
5. 获取用户输入的等分数量
使用Editor类的GetInteger方法获取用户输入的等分数量。
```csharp
PromptIntegerOptions pio = new PromptIntegerOptions("\nEnter number of divisions: ");
pio.AllowNegative = false;
pio.AllowZero = false;
pio.DefaultValue = 1;
PromptIntegerResult pir = ed.GetInteger(pio);
if (pir.Status != PromptStatus.OK) return;
int nDivisions = pir.Value;
```
6. 计算等分点坐标
使用Line类的GetClosestPointTo方法获取两条直线的交点,然后使用Vector3d类的GetNormal方法获取两条直线的交点的法向量。最后,使用Vector3d类的RotateBy方法计算等分点的坐标。
```csharp
Line ln1 = (Line)tr.GetObject(id1, OpenMode.ForRead);
Line ln2 = (Line)tr.GetObject(id2, OpenMode.ForRead);
Point3d intPt;
ln1.IntersectWith(ln2, Intersect.OnBothOperands, out intPt, IntPtr.Zero, IntPtr.Zero);
Vector3d dir1 = ln1.EndPoint - ln1.StartPoint;
Vector3d dir2 = ln2.EndPoint - ln2.StartPoint;
Vector3d normal = dir1.GetNormal(dir2);
double angle = 2.0 * Math.PI / nDivisions;
for (int i = 1; i < nDivisions; i++)
{
Point3d pt = intPt + normal.RotateBy(angle * i, Vector3d.ZAxis);
// TODO: Draw point or line here
}
```
7. 生成DLL文件
最后,编译代码生成DLL文件,并将它加载到AutoCAD中。
现在你的CAD二次开发.NET代码就完成了!用户在CAD中加载该DLL文件后,可以使用"MyCommand"命令选择两条直线并输入等分数量,然后生成相应等分线。
阅读全文
相关推荐

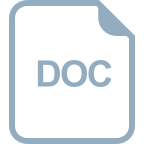











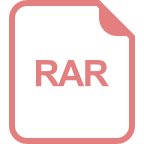

