#define _ _ACCOUNT_H_ _
时间: 2023-09-16 14:07:16 浏览: 46
这是一个预处理指令,用于防止头文件被重复包含。一般情况下,当一个头文件被多个源文件包含时,该头文件的内容会在每个源文件中重复定义,导致编译错误。使用预处理指令可以解决这个问题。在头文件的开头加上 #ifndef _ACCOUNT_H_ 和 #define _ACCOUNT_H_ 后,如果该头文件已经被包含过了,就不会再次包含了。在头文件的结尾加上 #endif 也是为了保险起见,防止出现意外情况。
相关问题
SkMaskFilter.h 代码
以下是SkMaskFilter.h的代码,您可以查看是否正确包含了SkBlurMaskFilter.h头文件:
```
#ifndef SkMaskFilter_DEFINED
#define SkMaskFilter_DEFINED
#include "include/core/SkRefCnt.h"
class SkMask;
class SkPaint;
class SkRect;
class SkString;
struct SkIRect;
class SkMatrix;
enum SkBlurStyle {
kNormal_SkBlurStyle, //!< fuzzy inside and outside
kSolid_SkBlurStyle, //!< solid inside, fuzzy outside
kOuter_SkBlurStyle, //!< nothing inside, fuzzy outside
kInner_SkBlurStyle, //!< fuzzy inside, nothing outside
kLastEnum_SkBlurStyle = kInner_SkBlurStyle
};
static constexpr int kBlurStyleCount = kLastEnum_SkBlurStyle + 1;
/** \class SkMaskFilter
SkMaskFilter is the base class for object that perform transformations on the mask before drawing
(in the maskFilter case) or after drawing (in the tableMaskFilter case).
*/
class SK_API SkMaskFilter : public SkRefCnt {
public:
~SkMaskFilter() override;
/** Given the input mask (generally from a SkGlyph), generate a new mask by applying the
filter's transform to it. The caller is responsible for freeing the returned mask.
@param dst storage for the new mask. It should be pre-allocated
@param src the original mask to be filtered
@param matrix transformation to apply to the mask, if the filter requires it
@param offset optional (may be nullptr) output parameter for the offset of the mask
relative to the original mask. The mask's bounds will be offset by this
amount relative to the glyph's left and top.
@return true if the resulting mask is not empty
*/
virtual bool filterMask(SkMask* dst, const SkMask& src, const SkMatrix& matrix,
SkIPoint* offset) const = 0;
/** Given the input blur radius, return the amount of additional space required in order to
account for the blur. This is used by the font cache to determine how much padding to add
when a glyph is added to the cache.
@param sigma The standard deviation of the desired Gaussian blur. Must be > 0.
@param style The style of the blur to apply.
@param bloatOutset If non-null, the resulting blur-outset will be added to this value.
@return The number of pixels of additional space required in each direction.
*/
virtual SkIRect filterBounds(const SkIRect& src, const SkMatrix& matrix,
SkBlurStyle style, SkScalar sigma) const;
/** Compute the blur radius for a given matrix and total sigma, taking into account this
filter's sigma and respectCTM setting.
@param matrix The matrix for the current draw operation.
@param totalSigma The total sigma to apply to the blur.
@return The sigma to use for this filter.
*/
virtual BlurRec computeBlurRec(const SkMatrix& matrix, SkScalar totalSigma) const = 0;
virtual void flatten(SkWriteBuffer&) const = 0;
int getFlattenedSize() const;
static sk_sp<SkMaskFilter> Deserialize(SkReadBuffer&);
static void RegisterFlattenables();
protected:
SkMaskFilter() = default;
private:
typedef SkRefCnt INHERITED;
friend class SkMaskFilterBase;
};
#endif
```
如果问题仍然存在,请提供更多上下文信息,以便我更好地帮助您。
编程题目 某校每位学生都要学习英语、语文、数学三门公共课程以及不同的专业课程。会计学专业要学习会计学和经济学两门课程,化学专业要学习有机化学和化学分析两门课程。编程序管理学生成绩,计算公共课的总分和平均分,以及所有课程的总成绩。 将每个类的声明与实现分别独立保存在与类同名的.h头文件和.cpp文件中。 comFinal类 Account类
好的,这是一个面向对象编程的问题,需要定义两个类:comFinal和Account。这里是comFinal的头文件和实现文件:
comFinal.h
```cpp
#ifndef COMFINAL_H
#define COMFINAL_H
#include <string>
#include <vector>
#include "Account.h"
class comFinal {
public:
comFinal(std::string name, std::string major); // 构造函数
~comFinal(); // 析构函数
void addCourse(std::string courseName); // 添加一门课程
void addScore(std::string courseName, double score); // 添加一门课程的成绩
double getPublicTotalScore() const; // 获取公共课总分
double getPublicAverageScore() const; // 获取公共课平均分
double getTotalScore() const; // 获取总成绩
private:
std::string name; // 学生姓名
std::string major; // 学生专业
std::vector<std::string> courses; // 所有课程名称
std::vector<double> scores; // 所有课程成绩
Account* account; // 学生账户
};
#endif
```
comFinal.cpp
```cpp
#include "comFinal.h"
comFinal::comFinal(std::string name, std::string major) {
this->name = name;
this->major = major;
this->account = new Account(); // 初始化学生账户
}
comFinal::~comFinal() {
delete account; // 释放学生账户内存
}
void comFinal::addCourse(std::string courseName) {
courses.push_back(courseName);
}
void comFinal::addScore(std::string courseName, double score) {
scores.push_back(score);
if (major == "会计学" && (courseName == "会计学" || courseName == "经济学")) {
account->addScore(score * 2); // 会计学专业的课程成绩要乘以2
} else if (major == "化学" && (courseName == "有机化学" || courseName == "化学分析")) {
account->addScore(score * 2); // 化学专业的课程成绩要乘以2
} else {
account->addScore(score);
}
}
double comFinal::getPublicTotalScore() const {
double totalScore = 0;
int count = 0;
for (int i = 0; i < courses.size(); ++i) {
if (courses[i] == "英语" || courses[i] == "语文" || courses[i] == "数学") {
totalScore += scores[i];
count++;
}
}
return totalScore;
}
double comFinal::getPublicAverageScore() const {
double totalScore = getPublicTotalScore();
int count = 0;
for (int i = 0; i < courses.size(); ++i) {
if (courses[i] == "英语" || courses[i] == "语文" || courses[i] == "数学") {
count++;
}
}
return totalScore / count;
}
double comFinal::getTotalScore() const {
return account->getTotalScore();
}
```
接下来是Account类的头文件和实现文件:
Account.h
```cpp
#ifndef ACCOUNT_H
#define ACCOUNT_H
class Account {
public:
Account(); // 构造函数
~Account(); // 析构函数
void addScore(double score); // 添加一门课程的成绩
double getTotalScore() const; // 获取总成绩
private:
double totalScore; // 总成绩
};
#endif
```
Account.cpp
```cpp
#include "Account.h"
Account::Account() {
totalScore = 0;
}
Account::~Account() {
}
void Account::addScore(double score) {
totalScore += score;
}
double Account::getTotalScore() const {
return totalScore;
}
```
这两个类的实现中,comFinal类负责管理每个学生的成绩和账户,Account类负责计算每个学生的总成绩。在comFinal类的addScore方法中,根据不同的专业和课程,将成绩放入对应的账户中。最终,通过调用comFinal类的getPublicTotalScore、getPublicAverageScore和getTotalScore方法,就可以计算出公共课的总分和平均分,以及所有课程的总成绩。
相关推荐
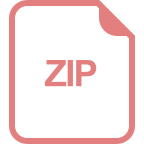
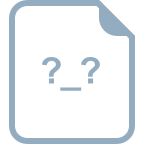
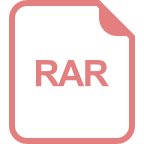













