string trim
时间: 2023-09-29 09:07:32 浏览: 44
字符串去除首尾空格的方法通常被称为 trim。在许多编程语言中,都有现成的函数或方法可以实现这个功能,以下是几种常见的实现方式:
1. 使用 trim() 方法
在 JavaScript、Java、Python、PHP 等语言中,都有现成的 trim() 方法可以去除字符串首尾空格。例如在 JavaScript 中:
```javascript
let str = " hello world ";
str = str.trim(); // "hello world"
```
2. 使用正则表达式
一些编程语言支持使用正则表达式来匹配字符串中的空格,并将其替换为空字符串。例如在 JavaScript 中:
```javascript
let str = " hello world ";
str = str.replace(/^\s+|\s+$/g, ''); // "hello world"
```
其中,`/^\s+|\s+$/g` 是一个正则表达式,表示匹配字符串开头和结尾的空格。
3. 手动实现
如果编程语言不支持 trim() 方法或正则表达式,我们也可以手动实现一个函数。例如在 C++ 中:
```cpp
#include <string>
std::string trim(std::string str) {
while (!str.empty() && std::isspace(str.front())) {
str.erase(0, 1);
}
while (!str.empty() && std::isspace(str.back())) {
str.pop_back();
}
return str;
}
```
该函数会移除字符串开头和结尾的空格,并返回处理后的字符串。
相关推荐
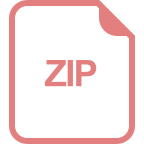














