编写一个字符加密的程序,加密规则是:从键盘输入一个大写或小写字母,将该字母按字母表上的顺序循环向后移3位,得到的字符即为密文,循环向后移时,Z的后面是A,B...。C语言
时间: 2024-05-20 18:16:48 浏览: 109
实现如下:
#include <stdio.h>
int main()
{
char ch, encrypted_ch;
printf("请输入一个大写或小写字母:");
scanf("%c", &ch);
if(ch >= 'A' && ch <= 'Z') // 大写字母
{
encrypted_ch = (ch - 'A' + 3) % 26 + 'A';
}
else if(ch >= 'a' && ch <= 'z') // 小写字母
{
encrypted_ch = (ch - 'a' + 3) % 26 + 'a';
}
else // 非字母字符
{
printf("输入的不是字母!\n");
return 0;
}
printf("加密后的密文为:%c\n", encrypted_ch);
return 0;
}
相关问题
编写一个字符加密的程序,加密规则是:从键盘输入一个大写或小写字母,将该字母按字母表上的顺序循环向后移3位,得到的字符即为密文,循环向后移时,Z的后面是A,B,…。
好的,我可以为您编写这样的程序。以下是 Python 代码示例:
```python
plain_text = input("请输入一个大写或小写字母:")
if plain_text.isalpha() and len(plain_text) == 1:
# 判断输入是否为字母且只有一个字符
# 将输入字符转换为小写
plain_text = plain_text.lower()
# 将字母表转换为列表
alphabet = list('abcdefghijklmnopqrstuvwxyz')
# 得到输入字符在字母表中的位置
position = alphabet.index(plain_text)
# 将位置加上3,并将结果对26取模,得到加密后的位置
cipher_position = (position + 3) % 26
# 根据加密后的位置得到加密后的字符
cipher_text = alphabet[cipher_position]
# 将加密后的字符转换为大写
cipher_text = cipher_text.upper()
print("加密后的字符为:", cipher_text)
else:
print("输入不符合要求!")
```
您可以将上述代码复制到 Python 解释器中运行,然后输入一个大写或小写字母,程序将输出加密后的字符。
c语言,编写一个字符加密的程序,加密规则是:从键盘输入一个大写或小写字母,将该字母按字母表上的顺序循环向后移三位,得到的字符即为密文,循环向后移时,z的后面是a,b
以下是C语言的代码实现:
```c
#include <stdio.h>
int main() {
char ch, encrypted;
printf("请输入一个大写或小写字母:");
scanf("%c", &ch);
if (ch >= 'a' && ch <= 'z') {
// 对小写字母进行加密
encrypted = (ch - 'a' + 3) % 26 + 'a';
} else if (ch >= 'A' && ch <= 'Z') {
// 对大写字母进行加密
encrypted = (ch - 'A' + 3) % 26 + 'A';
} else {
printf("输入的不是字母!\n");
return 0;
}
printf("加密后的字符为:%c\n", encrypted);
return 0;
}
```
运行程序后,会提示输入一个大写或小写字母,输入后程序会自动将该字母加密并输出。注意,如果输入的不是字母,则程序会提示输入错误。
阅读全文
相关推荐
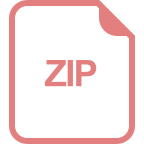
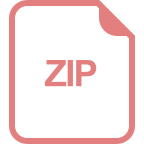
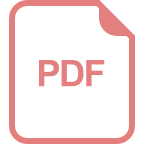












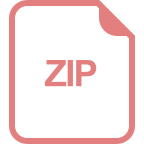
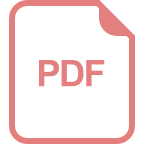