extend jframe
时间: 2023-08-13 13:11:50 浏览: 96
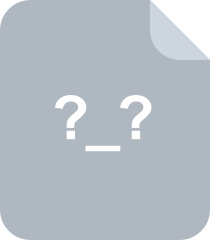
UserLogIn extends JFrame.java
在Java中,可以通过继承JFrame类来创建GUI应用程序窗口。JFrame类是Java Swing库中的一个类,它提供了创建GUI应用程序窗口的各种方法和属性。
下面是一个简单的示例代码,演示如何通过继承JFrame类创建一个简单的GUI应用程序窗口:
```
import javax.swing.JFrame;
public class MyFrame extends JFrame {
public MyFrame() {
setTitle("My Frame");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
MyFrame frame = new MyFrame();
}
}
```
在上面的代码中,我们创建了一个名为MyFrame的类,并继承了JFrame类。在构造函数中,我们设置了窗口的标题、大小、关闭操作和可见性。在main方法中,我们创建了一个MyFrame对象,这将显示GUI应用程序窗口。
当你运行这个程序时,你应该能够看到一个简单的GUI应用程序窗口,具有一个标题栏和一个空白的客户区域。你可以在这个窗口中添加各种GUI组件,例如按钮、标签、文本框等。
阅读全文
相关推荐
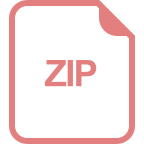
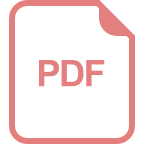


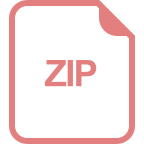
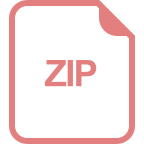