请用Java编写一个不需要界面,可以输出胜利棋子坐标的简易五子棋程序
时间: 2024-03-06 15:52:07 浏览: 104
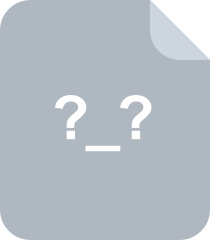
一个用java写的简单五子棋代码

好的,以下是一个简易的五子棋程序,可以在控制台输出胜利棋子的坐标:
```java
import java.util.Scanner;
public class FiveChess {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int[][] chessboard = new int[15][15]; // 棋盘
int player = 1; // 玩家编号,1表示黑子,2表示白子
int x, y; // 棋子坐标
boolean win = false; // 是否胜利的标记
while (!win) {
System.out.print("请输入玩家" + player + "的棋子坐标(以空格分隔):");
x = scanner.nextInt();
y = scanner.nextInt();
if (x < 0 || x > 14 || y < 0 || y > 14) {
System.out.println("坐标输入有误,请重新输入");
continue;
}
if (chessboard[x][y] != 0) {
System.out.println("该位置已经有棋子,请重新输入");
continue;
}
chessboard[x][y] = player; // 在棋盘上放置棋子
// 判断是否胜利
if (checkWin(chessboard, x, y, player)) {
System.out.println("玩家" + player + "获胜");
win = true;
}
// 切换玩家
player = player == 1 ? 2 : 1;
}
}
// 判断是否胜利
private static boolean checkWin(int[][] chessboard, int x, int y, int player) {
int count = 1; // 连续棋子的数量
int i, j;
// 横向判断
i = x;
j = y - 1;
while (j >= 0 && chessboard[i][j] == player) {
count++;
j--;
}
j = y + 1;
while (j <= 14 && chessboard[i][j] == player) {
count++;
j++;
}
if (count >= 5) {
return true;
}
// 纵向判断
count = 1;
i = x - 1;
j = y;
while (i >= 0 && chessboard[i][j] == player) {
count++;
i--;
}
i = x + 1;
while (i <= 14 && chessboard[i][j] == player) {
count++;
i++;
}
if (count >= 5) {
return true;
}
// 斜向(左上-右下)判断
count = 1;
i = x - 1;
j = y - 1;
while (i >= 0 && j >= 0 && chessboard[i][j] == player) {
count++;
i--;
j--;
}
i = x + 1;
j = y + 1;
while (i <= 14 && j <= 14 && chessboard[i][j] == player) {
count++;
i++;
j++;
}
if (count >= 5) {
return true;
}
// 斜向(左下-右上)判断
count = 1;
i = x - 1;
j = y + 1;
while (i >= 0 && j <= 14 && chessboard[i][j] == player) {
count++;
i--;
j++;
}
i = x + 1;
j = y - 1;
while (i <= 14 && j >= 0 && chessboard[i][j] == player) {
count++;
i++;
j--;
}
if (count >= 5) {
return true;
}
return false;
}
}
```
程序运行后,会提示玩家输入棋子坐标,例如:3 4 表示在第4行第5列(从0开始计数)放置棋子。如果某个玩家获胜,则会在控制台输出相应的提示信息,并退出程序。
阅读全文
相关推荐
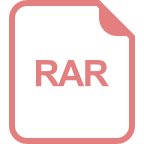
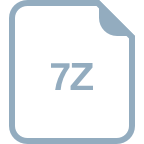
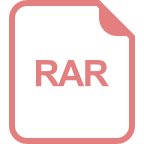
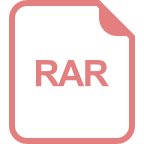
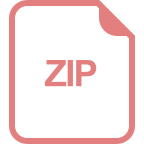
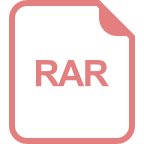
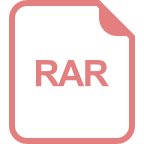
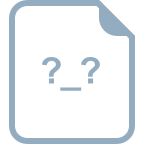
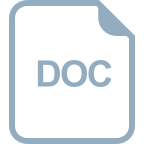
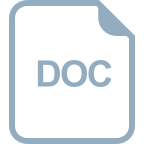

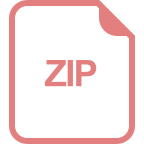
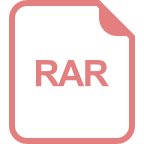
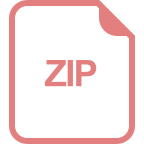
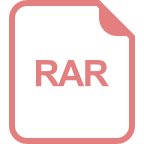
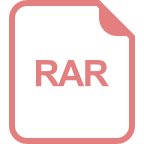
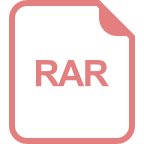