我需要一个有两个选择题各有四个选项的android页面 要有xml和java代码 全部的完整代码
时间: 2024-05-05 10:17:42 浏览: 114
XML代码:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/question_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="What is the capital of France?" />
<RadioGroup
android:id="@+id/answer_radiogroup"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:orientation="vertical">
<RadioButton
android:id="@+id/answer1_radiobutton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="London" />
<RadioButton
android:id="@+id/answer2_radiobutton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Paris" />
<RadioButton
android:id="@+id/answer3_radiobutton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Madrid" />
<RadioButton
android:id="@+id/answer4_radiobutton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Rome" />
</RadioGroup>
<Button
android:id="@+id/submit_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:text="Submit" />
</LinearLayout>
```
Java代码:
```
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.TextView;
import android.widget.Toast;
public class QuizActivity extends AppCompatActivity {
private TextView mQuestionTextView;
private RadioGroup mAnswerRadioGroup;
private RadioButton mAnswer1RadioButton;
private RadioButton mAnswer2RadioButton;
private RadioButton mAnswer3RadioButton;
private RadioButton mAnswer4RadioButton;
private Button mSubmitButton;
private int mCorrectAnswerIndex = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_quiz);
mQuestionTextView = findViewById(R.id.question_textview);
mAnswerRadioGroup = findViewById(R.id.answer_radiogroup);
mAnswer1RadioButton = findViewById(R.id.answer1_radiobutton);
mAnswer2RadioButton = findViewById(R.id.answer2_radiobutton);
mAnswer3RadioButton = findViewById(R.id.answer3_radiobutton);
mAnswer4RadioButton = findViewById(R.id.answer4_radiobutton);
mSubmitButton = findViewById(R.id.submit_button);
mQuestionTextView.setText("What is the capital of France?");
mAnswer1RadioButton.setText("London");
mAnswer2RadioButton.setText("Paris");
mAnswer3RadioButton.setText("Madrid");
mAnswer4RadioButton.setText("Rome");
mSubmitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int selectedAnswerIndex = mAnswerRadioGroup.getCheckedRadioButtonId();
if (selectedAnswerIndex == -1) {
Toast.makeText(QuizActivity.this, "Please select an answer", Toast.LENGTH_SHORT).show();
} else if (selectedAnswerIndex == mAnswer1RadioButton.getId()) {
Toast.makeText(QuizActivity.this, "Incorrect", Toast.LENGTH_SHORT).show();
} else if (selectedAnswerIndex == mAnswer2RadioButton.getId()) {
Toast.makeText(QuizActivity.this, "Correct", Toast.LENGTH_SHORT).show();
} else if (selectedAnswerIndex == mAnswer3RadioButton.getId()) {
Toast.makeText(QuizActivity.this, "Incorrect", Toast.LENGTH_SHORT).show();
} else if (selectedAnswerIndex == mAnswer4RadioButton.getId()) {
Toast.makeText(QuizActivity.this, "Incorrect", Toast.LENGTH_SHORT).show();
}
}
});
}
}
```
阅读全文
相关推荐
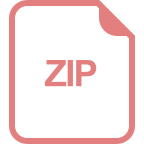

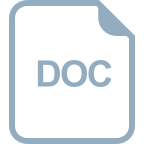
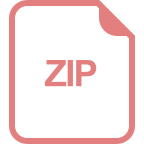
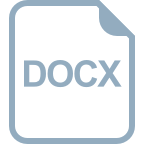
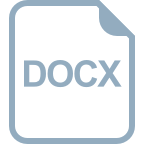
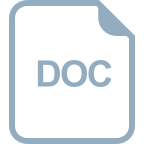
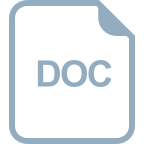
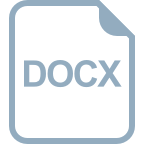
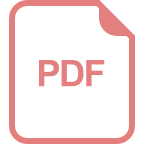
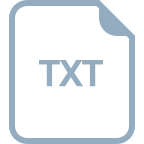
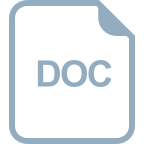
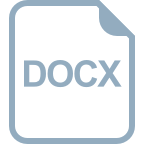
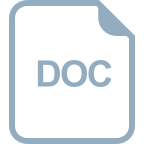
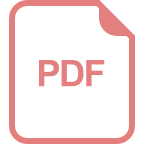
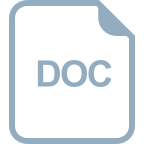